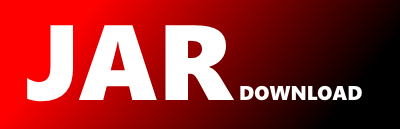
com.github.becausetesting.commonswindow.utils.ui.JPaneUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-window Show documentation
Show all versions of commons-window Show documentation
A common libraries used for windows framework.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy