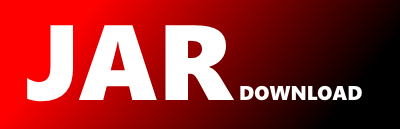
bc.ui.swing.lists.StripeList Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Benny Lutati.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package bc.ui.swing.lists;
import bc.ui.swing.models.GenericListModel;
import bc.ui.swing.visuals.Visual;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.util.LinkedList;
import java.util.List;
import javax.swing.JList;
import javax.swing.JViewport;
import javax.swing.event.ListSelectionListener;
/**
*
* @author bennyl
*/
public class StripeList extends javax.swing.JPanel {
private Color evenBackColor = new Color(245, 245, 245);
private Color evenForeColor = new Color(51, 51, 51);
private Color oddBackColor = new Color(215, 215, 215);
private Color oddForeColor = new Color(71, 71, 71);
private final StripeListItemRenderer itemRenderer;
/** Creates new form StripeList */
public StripeList() {
initComponents();
list.setFixedCellHeight(24);
scroll.setViewport(new StripeViewPort());
scroll.setViewportView(list);
itemRenderer = new StripeListItemRenderer();
list.setCellRenderer(itemRenderer);
}
public void addSelectionListner(ListSelectionListener listener) {
list.getSelectionModel().addListSelectionListener(listener);
}
public void setItems(LinkedList visuals) {
GenericListModel data = new GenericListModel();
data.setInnerList(visuals);
list.setModel(data);
}
public void remove(Visual item){
GenericListModel data = (GenericListModel) list.getModel();
data.remove(item);
}
@Override
public void setFont(Font font) {
if (itemRenderer != null) {
itemRenderer.setFont(font);
}
}
@Override
public Font getFont() {
if (itemRenderer != null) {
return itemRenderer.getFont();
}
return super.getFont();
}
public JList getList() {
return list;
}
public List getSelectedItems() {
LinkedList ret = new LinkedList();
for (Object l : list.getSelectedValues()) {
ret.add((Visual) l);
}
return ret;
}
public Color getEvenBackColor() {
return evenBackColor;
}
public void setEvenBackColor(Color evenBackColor) {
this.evenBackColor = evenBackColor;
itemRenderer.setEvenBackColor(evenBackColor);
}
public Color getEvenForeColor() {
return evenForeColor;
}
public void setEvenForeColor(Color evenForeColor) {
this.evenForeColor = evenForeColor;
itemRenderer.setEvenForeColor(evenForeColor);
}
public Color getOddBackColor() {
return oddBackColor;
}
public void setOddBackColor(Color oddBackColor) {
this.oddBackColor = oddBackColor;
itemRenderer.setOddBackColor(oddBackColor);
}
public Color getOddForeColor() {
return oddForeColor;
}
public void setOddForeColor(Color oddForeColor) {
this.oddForeColor = oddForeColor;
itemRenderer.setOddForeColor(oddForeColor);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
scroll = new javax.swing.JScrollPane();
list = new javax.swing.JList();
setLayout(new java.awt.BorderLayout());
scroll.setBorder(null);
list.setModel(new javax.swing.AbstractListModel() {
String[] strings = { "Item 1", "Item 2", "Item 3", "Item 4", "Item 5" };
public int getSize() { return strings.length; }
public Object getElementAt(int i) { return strings[i]; }
});
list.setOpaque(false);
scroll.setViewportView(list);
add(scroll, java.awt.BorderLayout.CENTER);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JList list;
private javax.swing.JScrollPane scroll;
// End of variables declaration//GEN-END:variables
private Color getRowColor(int i) {
if (i % 2 == 0) {
return evenBackColor;
}
return oddBackColor;
}
private class StripeViewPort extends JViewport {
@Override
protected void paintComponent(Graphics g) {
paintStripedBackground(g);
//super.paintComponent(g);
}
private void paintStripedBackground(Graphics g) {
// get the row index at the top of the clip bounds (the first row
// to paint).
int rowAtPoint = list.locationToIndex(g.getClipBounds().getLocation());
// get the y coordinate of the first row to paint. if there are no
// rows in the table, start painting at the top of the supplied
// clipping bounds.
int topY = rowAtPoint < 0
? g.getClipBounds().y : list.getCellBounds(rowAtPoint, 0).y;
// create a counter variable to hold the current row. if there are no
// rows in the table, start the counter at 0.
int currentRow = rowAtPoint < 0 ? 0 : rowAtPoint;
while (topY < g.getClipBounds().y + g.getClipBounds().height) {
int bottomY = topY + list.getFixedCellHeight();
g.setColor(getRowColor(currentRow));
g.fillRect(g.getClipBounds().x, topY, g.getClipBounds().width, bottomY);
topY = bottomY;
currentRow++;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy