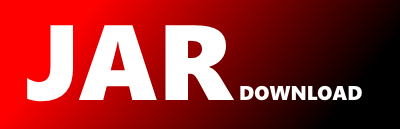
bc.ui.swing.tables.ScrollableStripeTable Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Benny Lutati.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package bc.ui.swing.tables;
import java.awt.Color;
import java.util.Enumeration;
import javax.swing.JFrame;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.TableColumnModelEvent;
import javax.swing.event.TableColumnModelListener;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableColumn;
import javax.swing.table.TableModel;
/**
*
* @author bennyl
*/
public class ScrollableStripeTable extends javax.swing.JPanel {
private static final StripeTableHeader HEADER_RENDERER = new StripeTableHeader();
/** Creates new form ScrollableStripeTable */
public ScrollableStripeTable() {
initComponents();
addColumnListener();
TableModel model = table.getModel();
table.setModel(new DefaultTableModel());
table.setModel(model);
}
@Override
public void setForeground(Color fg) {
if (table != null) {
table.setForeground(fg);
} else {
super.setForeground(fg);
}
}
@Override
public Color getForeground() {
if (table != null) {
return table.getForeground();
} else {
return super.getForeground();
}
}
public void setOddRowColor(Color oddRowColor) {
table.setOddRowColor(oddRowColor);
}
public void setEvenRowColor(Color evenRowColor) {
table.setEvenRowColor(evenRowColor);
}
public Color getOddRowColor() {
return table.getOddRowColor();
}
public Color getEvenRowColor() {
return table.getEvenRowColor();
}
public void setModel(TableModel dataModel) {
table.setModel(dataModel);
}
public TableModel getModel() {
return table.getModel();
}
private void addColumnListener() {
table.getColumnModel().addColumnModelListener(new TableColumnModelListener() {
@Override
public void columnAdded(TableColumnModelEvent e) {
final Enumeration cols = table.getColumnModel().getColumns();
while (cols.hasMoreElements()) {
TableColumn col = cols.nextElement();
col.setHeaderRenderer(HEADER_RENDERER);
}
}
@Override
public void columnRemoved(TableColumnModelEvent e) {
// throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void columnMoved(TableColumnModelEvent e) {
// throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void columnMarginChanged(ChangeEvent e) {
// throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void columnSelectionChanged(ListSelectionEvent e) {
// throw new UnsupportedOperationException("Not supported yet.");
}
});
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
frame.setContentPane(new ScrollableStripeTable());
frame.pack();
frame.setVisible(true);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane();
table = new bc.ui.swing.tables.StripeTable();
setLayout(new java.awt.BorderLayout());
jScrollPane1.setBorder(null);
table.setForeground(new java.awt.Color(255, 255, 255));
table.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{"bla", "bla", "bla", "bvlsdf"},
{"dsfsdfgdf", "frdgsdfbsdfg", "edfgsdfsdf", "dfgbsdfgsd"},
{"sdfgsdfgs", "fgsdfgsdf", "efgsdfg", "fgsdfg"},
{"dfgsdfgsdfhdsf", "fghdrtywer", "rtyerytwert", null}
},
new String [] {
"Title 1", "Title 2", "Title 3", "Title 4"
}
));
table.setIntercellSpacing(new java.awt.Dimension(0, 0));
table.setShowHorizontalLines(false);
table.setShowVerticalLines(false);
jScrollPane1.setViewportView(table);
add(jScrollPane1, java.awt.BorderLayout.CENTER);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JScrollPane jScrollPane1;
private bc.ui.swing.tables.StripeTable table;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy