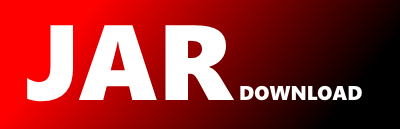
io.datatree.dom.DeepCloner Maven / Gradle / Ivy
/**
* This software is licensed under the Apache 2 license, quoted below.
*
* Copyright 2017 Andras Berkes [[email protected]]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.datatree.dom;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.net.Inet4Address;
import java.net.Inet6Address;
import java.net.InetAddress;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
/**
* Recursive deep cloner utility.
*
* @author Andras Berkes [[email protected]]
*/
public class DeepCloner {
// --- IMMUTABLE CLASSES ---
/**
* List of immutable objects (Long, Integer, String, etc.).
*/
private static final HashSet> immutableClasses = new HashSet<>(64);
static {
addImmutableClass(String.class, Boolean.class, BigDecimal.class, BigInteger.class, InetAddress.class,
Inet4Address.class, Inet6Address.class, Long.class, Double.class, Float.class, Short.class, Byte.class,
Integer.class);
}
// --- PRIVATE CONSTRUCTOR ---
private DeepCloner() {
}
/**
* Adds immutable classes (for faster cloning). This method is not
* thread-safe!
*
* @param immutableClass
* classes
*/
public static final void addImmutableClass(Class>... immutableClass) {
immutableClasses.addAll(Arrays.asList(immutableClass));
}
/**
* Removes mutable classes from internal Set. This method is not
* thread-safe!
*
* @param mutableClass
* classes
*/
public static final void removeImmutableClass(Class>... mutableClass) {
immutableClasses.removeAll(Arrays.asList(mutableClass));
}
// --- VALUE / MAP / LIST / SET CLONER ---
@SuppressWarnings("unchecked")
public static final Object clone(Object from) throws Exception {
// Cloning "null" value
if (from == null) {
return null;
}
// Cloning Maps
if (from instanceof Map) {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy