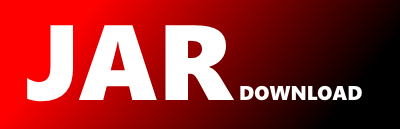
io.datatree.dom.builtin.AbstractAdapter Maven / Gradle / Ivy
/**
* This software is licensed under the Apache 2 license, quoted below.
*
* Copyright 2017 Andras Berkes [[email protected]]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.datatree.dom.builtin;
import java.util.Map;
import java.util.function.Consumer;
import io.datatree.dom.Config;
import io.datatree.dom.TreeReader;
import io.datatree.dom.TreeWriter;
import io.datatree.dom.WriterFunction;
/**
* Reader / writer class.
*
* @author Andras Berkes [[email protected]]
*/
public abstract class AbstractAdapter implements TreeReader, TreeWriter {
@SafeVarargs
@SuppressWarnings({ "unchecked" })
protected static final boolean tryToAddSerializers(String consumerClass, T... factories) {
boolean allInstalled = true;
for (T factory : factories) {
try {
((Consumer) Class.forName(consumerClass).newInstance()).accept(factory);
} catch (Throwable ignored) {
// Classes aren't available
allInstalled = false;
}
}
return allInstalled;
}
protected static final byte[] toBinary(Object value, Object meta, boolean insertMeta, WriterFunction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy