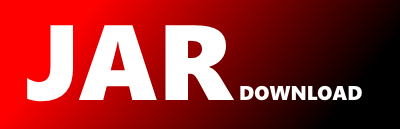
org.unique.commons.io.PropUtil Maven / Gradle / Ivy
/**
* Copyright (c) 2014-2015, biezhi 王爵 ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.unique.commons.io;
import java.io.IOException;
import java.io.InputStream;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.unique.commons.utils.CollectionUtil;
/**
* properties file util
* @author biezhi
* @since 1.0
*/
public class PropUtil {
private static final Logger logger = LoggerFactory.getLogger(PropUtil.class);
/**
* 根据文件名读取properties文件
*/
public static Properties getProperty(String resourceName) {
InputStream in = null;
Properties props = new Properties();
try {
in = Thread.currentThread().getContextClassLoader().getResourceAsStream(resourceName);
if (in != null) {
props.load(in);
}
} catch (IOException e) {
logger.error("加载属性文件出错!", e);
} finally {
IOUtil.closeQuietly(in);
}
return props;
}
/**
* //TODO 根据文件名读取map数据
*/
public static Map getPropertyMap(String resourceName) {
InputStream in = null;
Map map = CollectionUtil.newHashMap();
try {
in = Thread.currentThread().getContextClassLoader().getResourceAsStream(resourceName);
if (null == in) {
throw new RuntimeException("没有找到资源文件 [" + resourceName + "]");
}
Properties prop = new Properties();
prop.load(in);
Set> set = prop.entrySet();
Iterator> it = set.iterator();
while (it.hasNext()) {
Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy