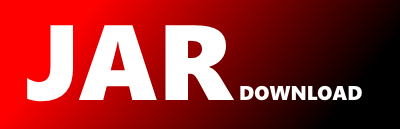
org.bk.aws.model.Result Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-sdk2-reactive-messaging Show documentation
Show all versions of aws-sdk2-reactive-messaging Show documentation
Generate a reactive stream from AWS SNS/SQS
The newest version!
package org.bk.aws.model;
/**
* A type to hold the output of a computation. If a computation is successful, it returns the result, for failure it returns the cause of failure.
*
* To be used this way:
* For a case where the computation is successful:
*
* Result result = Result.of(() -> {
* return "hello";
* });
*
* result.isSucess() == true;
*
*
* For the case where the computation results in a failure:
*
*
* Result result = Result.of(() -> {
* throw new RuntimeException("failed");
* });
*
*
* result.isFailure() == true;
* (result.getCause() instanceof Throwable) == true;
*
*
* This is specifically created for the stream processing flow where it is important that any computation
* not throw an exception as it then breaks out of the reactive streams pipeline, Result will wrap it up
* to be handled in a subsequent step of the pipeline.
*
* @param
*/
public interface Result {
boolean isSuccess();
boolean isFailure();
T get();
Throwable getCause();
static Result success(T body) {
return new Success<>(body);
}
static Result failure(Throwable t) {
return new Failure<>(t);
}
static Result of(ThrowingSupplier supplier) {
try {
return new Success<>(supplier.get());
} catch (Throwable throwable) {
return new Failure<>(throwable);
}
}
class Success implements Result {
private final T value;
public Success(T value) {
this.value = value;
}
@Override
public boolean isSuccess() {
return true;
}
@Override
public boolean isFailure() {
return false;
}
@Override
public T get() {
return value;
}
@Override
public Throwable getCause() {
return null;
}
}
class Failure implements Result {
private final Throwable cause;
public Failure(Throwable cause) {
this.cause = cause;
}
@Override
public boolean isSuccess() {
return false;
}
@Override
public boolean isFailure() {
return true;
}
@Override
public T get() {
throw new RuntimeException(this.getCause());
}
@Override
public Throwable getCause() {
return cause;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy