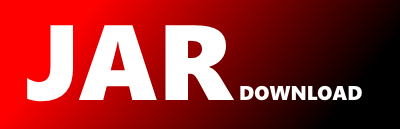
me.chanjar.weixin.channel.util.XmlUtils Maven / Gradle / Ivy
package me.chanjar.weixin.channel.util;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.ser.ToXmlGenerator;
import java.io.IOException;
import java.io.InputStream;
import lombok.extern.slf4j.Slf4j;
/**
* Xml序列化/反序列化工具类
*
* @author Zeyes
*/
@Slf4j
public class XmlUtils {
private static final XmlMapper XML_MAPPER = new XmlMapper();
static {
XML_MAPPER.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
XML_MAPPER.disable(DeserializationFeature.FAIL_ON_IGNORED_PROPERTIES);
// 带有xml文件头,
XML_MAPPER.disable(ToXmlGenerator.Feature.WRITE_XML_DECLARATION);
}
private XmlUtils() {
}
/**
* 对象序列化
*
* @param obj 对象
* @return json
*/
public static String encode(Object obj) {
try {
return XML_MAPPER.writeValueAsString(obj);
} catch (IOException e) {
log.error("encode(Object)", e);
}
return null;
}
/**
* 对象序列化
*
* @param objectMapper ObjectMapper
* @param obj obj
* @return json
*/
public static String encode(ObjectMapper objectMapper, Object obj) {
try {
return objectMapper.writeValueAsString(obj);
} catch (IOException e) {
log.error("encode(Object)", e);
}
return null;
}
/**
* 将xml反序列化成对象
*
* @param xml xml
* @param valueType Class
* @return T
*/
public static T decode(String xml, Class valueType) {
if (xml == null || xml.length() <= 0) {
return null;
}
try {
return XML_MAPPER.readValue(xml, valueType);
} catch (IOException e) {
log.info("decode(String, Class)", e);
}
return null;
}
/**
* 将xml反序列化为对象
*
* @param xml xml
* @param typeReference TypeReference
* @return T
*/
public static T decode(String xml, TypeReference typeReference) {
try {
return (T) XML_MAPPER.readValue(xml, typeReference);
} catch (IOException e) {
log.info("decode(String, TypeReference)", e);
}
return null;
}
/**
* 将xml反序列化为对象
*
* @param is InputStream
* @param valueType Class
* @return T
*/
public static T decode(InputStream is, Class valueType) {
try {
return (T) XML_MAPPER.readValue(is, valueType);
} catch (IOException e) {
log.info("decode(InputStream, Class)", e);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy