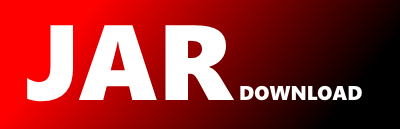
me.chanjar.weixin.common.util.XmlUtils Maven / Gradle / Ivy
The newest version!
package me.chanjar.weixin.common.util;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import me.chanjar.weixin.common.error.WxRuntimeException;
import org.dom4j.*;
import org.dom4j.io.SAXReader;
import org.dom4j.tree.DefaultText;
import org.xml.sax.SAXException;
import java.io.StringReader;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
/**
*
* XML转换工具类.
* Created by Binary Wang on 2018/11/4.
*
*
* @author Binary Wang
*/
public class XmlUtils {
public static Map xml2Map(String xmlString) {
Map map = new HashMap<>(16);
try {
SAXReader saxReader = new SAXReader();
saxReader.setFeature("http://apache.org/xml/features/disallow-doctype-decl", true);
saxReader.setFeature("http://javax.xml.XMLConstants/feature/secure-processing", true);
saxReader.setFeature("http://xml.org/sax/features/external-parameter-entities", false);
saxReader.setFeature("http://xml.org/sax/features/external-general-entities", false);
saxReader.setFeature("http://apache.org/xml/features/nonvalidating/load-external-dtd", false);
Document doc = saxReader.read(new StringReader(xmlString));
Element root = doc.getRootElement();
List elements = root.elements();
for (Element element : elements) {
String elementName = element.getName();
if (map.containsKey(elementName)) {
if (map.get(elementName) instanceof List) {
((List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy