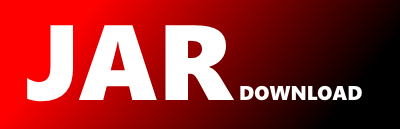
me.chanjar.weixin.mp.bean.pay.request.WxPayRefundRequest Maven / Gradle / Ivy
package me.chanjar.weixin.mp.bean.pay.request;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import me.chanjar.weixin.common.annotation.Required;
/**
*
* 微信支付-申请退款请求参数
* 注释中各行每个字段描述对应如下:
*
字段名
* 变量名
* 是否必填
* 类型
* 示例值
* 描述
*
*
* @author binarywang(Binary Wang)
* Created by Binary Wang on 2016-10-08.
*/
@XStreamAlias("xml")
public class WxPayRefundRequest {
/**
* * 公众账号ID * appid * 是 * String(32) * wx8888888888888888 * 微信分配的公众账号ID(企业号corpid即为此appId) **/ @XStreamAlias("appid") private String appid; /** *
* 商户号 * mch_id * 是 * String(32) * 1900000109 * 微信支付分配的商户号 **/ @XStreamAlias("mch_id") private String mchId; /** *
* 设备号 * device_info * 否 * String(32) * 13467007045764 * 终端设备号 **/ @XStreamAlias("device_info") private String deviceInfo; /** *
* 随机字符串 * nonce_str * 是 * String(32) * 5K8264ILTKCH16CQ2502SI8ZNMTM67VS * 随机字符串,不长于32位。推荐随机数生成算法 **/ @XStreamAlias("nonce_str") private String nonceStr; /** *
* 签名 * sign * 是 * String(32) * C380BEC2BFD727A4B6845133519F3AD6 * 签名,详见签名生成算法 **/ @XStreamAlias("sign") private String sign; /** *
* 微信订单号 * transaction_id * 跟out_trade_no二选一 * String(28) * 1217752501201400000000000000 * 微信生成的订单号,在支付通知中有返回 **/ @XStreamAlias("transaction_id") private String transactionId; /** *
* 商户订单号 * out_trade_no * 跟transaction_id二选一 * String(32) * 1217752501201400000000000000 * 商户侧传给微信的订单号 **/ @XStreamAlias("out_trade_no") private String outTradeNo; /** *
* 商户退款单号 * out_refund_no * 是 * String(32) * 1217752501201400000000000000 * 商户系统内部的退款单号,商户系统内部唯一,同一退款单号多次请求只退一笔 **/ @Required @XStreamAlias("out_refund_no") private String outRefundNo; /** *
* 订单金额 * total_fee * 是 * Int * 100 * 订单总金额,单位为分,只能为整数,详见支付金额 **/ @Required @XStreamAlias("total_fee") private Integer totalFee; /** *
* 退款金额 * refund_fee * 是 * Int * 100 * 退款总金额,订单总金额,单位为分,只能为整数,详见支付金额 **/ @Required @XStreamAlias("refund_fee") private Integer refundFee; /** *
* 货币种类 * refund_fee_type * 否 * String(8) * CNY * 货币类型,符合ISO 4217标准的三位字母代码,默认人民币:CNY,其他值列表详见货币类型 **/ @XStreamAlias("refund_fee_type") private String refundFeeType; /** *
* 操作员 * op_user_id * 是 * String(32) * 1900000109 * 操作员帐号, 默认为商户号 **/ //@Required @XStreamAlias("op_user_id") private String opUserId; /** *
* 退款资金来源 * refund_account * 否 * String(30) * REFUND_SOURCE_RECHARGE_FUNDS * 仅针对老资金流商户使用, *
© 2015 - 2025 Weber Informatics LLC | Privacy Policy