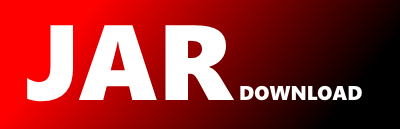
com.github.binarywang.wxpay.bean.result.WxPayOrderNotifyResult Maven / Gradle / Ivy
package com.github.binarywang.wxpay.bean.result;
import com.github.binarywang.wxpay.bean.WxPayOrderNotifyCoupon;
import com.github.binarywang.wxpay.converter.WxPayOrderNotifyResultConverter;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import me.chanjar.weixin.common.util.BeanUtils;
import me.chanjar.weixin.common.util.ToStringUtils;
import me.chanjar.weixin.common.util.xml.XStreamInitializer;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
/**
* 支付结果通用通知 ,文档见:https://pay.weixin.qq.com/wiki/doc/api/jsapi.php?chapter=9_7
*
* @author aimilin6688
* @since 2.5.0
*/
@XStreamAlias("xml")
public class WxPayOrderNotifyResult extends WxPayBaseResult implements Serializable {
private static final long serialVersionUID = 5389718115223345496L;
/**
*
* 字段名:设备号
* 变量名:device_info
* 是否必填:否
* 类型:String(32)
* 示例值:013467007045764
* 描述:微信支付分配的终端设备号,
*
*/
@XStreamAlias("device_info")
private String deviceInfo;
/**
*
* 字段名:用户标识
* 变量名:openid
* 是否必填:是
* 类型:String(128)
* 示例值:wxd930ea5d5a258f4f
* 描述:用户在商户appid下的唯一标识
*
*/
@XStreamAlias("openid")
private String openid;
/**
*
* 字段名:是否关注公众账号
* 变量名:is_subscribe
* 是否必填:否
* 类型:String(1)
* 示例值:Y
* 描述:用户是否关注公众账号,Y-关注,N-未关注,仅在公众账号类型支付有效
*
*/
@XStreamAlias("is_subscribe")
private String isSubscribe;
/**
*
* 字段名:用户子标识
* 变量名:sub_openid
* 是否必填:是
* 类型:String(128)
* 示例值:wxd930ea5d5a258f4f
* 描述:用户在子商户appid下的唯一标识
*
*/
@XStreamAlias("sub_openid")
private String subOpenid;
/**
*
* 字段名:是否关注子公众账号
* 变量名:sub_is_subscribe
* 是否必填:否
* 类型:String(1)
* 示例值:Y
* 描述:用户是否关注子公众账号,Y-关注,N-未关注,仅在公众账号类型支付有效
*
*/
@XStreamAlias("sub_is_subscribe")
private String subIsSubscribe;
/**
*
* 字段名:交易类型
* 变量名:trade_type
* 是否必填:是
* 类型:String(16)
* 示例值:JSAPI
* JSA描述:PI、NATIVE、APP
*
*/
@XStreamAlias("trade_type")
private String tradeType;
/**
*
* 字段名:付款银行
* 变量名:bank_type
* 是否必填:是
* 类型:String(16)
* 示例值:CMC
* 描述:银行类型,采用字符串类型的银行标识,银行类型见银行列表
*
*/
@XStreamAlias("bank_type")
private String bankType;
/**
*
* 字段名:订单金额
* 变量名:total_fee
* 是否必填:是
* 类型:Int
* 示例值:100
* 描述:订单总金额,单位为分
*
*/
@XStreamAlias("total_fee")
private Integer totalFee;
/**
*
* 字段名:应结订单金额
* 变量名:settlement_total_fee
* 是否必填:否
* 类型:Int
* 示例值:100
* 描述:应结订单金额=订单金额-非充值代金券金额,应结订单金额<=订单金额。
*
*/
@XStreamAlias("settlement_total_fee")
private Integer settlementTotalFee;
/**
*
* 字段名:货币种类
* 变量名:fee_type
* 是否必填:否
* 类型:String(8)
* 示例值:CNY
* 描述:货币类型,符合ISO4217标准的三位字母代码,默认人民币:CNY,其他值列表详见货币类型
*
*/
@XStreamAlias("fee_type")
private String feeType;
/**
*
* 字段名:现金支付金额
* 变量名:cash_fee
* 是否必填:是
* 类型:Int
* 示例值:100
* 描述:现金支付金额订单现金支付金额,详见支付金额
*
*/
@XStreamAlias("cash_fee")
private Integer cashFee;
/**
*
* 字段名:现金支付货币类型
* 变量名:cash_fee_type
* 是否必填:否
* 类型:String(16)
* 示例值:CNY
* 描述:货币类型,符合ISO4217标准的三位字母代码,默认人民币:CNY,其他值列表详见货币类型
*
*/
@XStreamAlias("cash_fee_type")
private String cashFeeType;
/**
*
* 字段名:总代金券金额
* 变量名:coupon_fee
* 是否必填:否
* 类型:Int
* 示例值:10
* 描述:代金券金额<=订单金额,订单金额-代金券金额=现金支付金额,详见支付金额
*
*/
@XStreamAlias("coupon_fee")
private Integer couponFee;
/**
*
* 字段名:代金券使用数量
* 变量名:coupon_count
* 是否必填:否
* 类型:Int
* 示例值:1
* 描述:代金券使用数量
*
*/
@XStreamAlias("coupon_count")
private Integer couponCount;
private List couponList;
/**
*
* 字段名:微信支付订单号
* 变量名:transaction_id
* 是否必填:是
* 类型:String(32)
* 示例值:1217752501201407033233368018
* 描述:微信支付订单号
*
*/
@XStreamAlias("transaction_id")
private String transactionId;
/**
*
* 字段名:商户订单号
* 变量名:out_trade_no
* 是否必填:是
* 类型:String(32)
* 示例值:1212321211201407033568112322
* 描述:商户系统的订单号,与请求一致。
*
*/
@XStreamAlias("out_trade_no")
private String outTradeNo;
/**
*
* 字段名:商家数据包
* 变量名:attach
* 是否必填:否
* 类型:String(128)
* 示例值:123456
* 描述:商家数据包,原样返回
*
*/
@XStreamAlias("attach")
private String attach;
/**
*
* 字段名:支付完成时间
* 变量名:time_end
* 是否必填:是
* 类型:String(14)
* 示例值:20141030133525
* 描述:支付完成时间,格式为yyyyMMddHHmmss,如2009年12月25日9点10分10秒表示为20091225091010。其他详见时间规则
*
*/
@XStreamAlias("time_end")
private String timeEnd;
public static WxPayOrderNotifyResult fromXML(String xmlString) {
XStream xstream = XStreamInitializer.getInstance();
xstream.processAnnotations(WxPayOrderNotifyResult.class);
xstream.registerConverter(new WxPayOrderNotifyResultConverter(xstream.getMapper(), xstream.getReflectionProvider()));
WxPayOrderNotifyResult result = (WxPayOrderNotifyResult) xstream.fromXML(xmlString);
result.setXmlString(xmlString);
return result;
}
public Integer getCouponCount() {
return couponCount;
}
public void setCouponCount(Integer couponCount) {
this.couponCount = couponCount;
}
public List getCouponList() {
return couponList;
}
public void setCouponList(List couponList) {
this.couponList = couponList;
}
public String getDeviceInfo() {
return deviceInfo;
}
public void setDeviceInfo(String deviceInfo) {
this.deviceInfo = deviceInfo;
}
public String getOpenid() {
return openid;
}
public void setOpenid(String openid) {
this.openid = openid;
}
public String getIsSubscribe() {
return isSubscribe;
}
public void setIsSubscribe(String isSubscribe) {
this.isSubscribe = isSubscribe;
}
public String getTradeType() {
return tradeType;
}
public void setTradeType(String tradeType) {
this.tradeType = tradeType;
}
public String getBankType() {
return bankType;
}
public void setBankType(String bankType) {
this.bankType = bankType;
}
public Integer getTotalFee() {
return totalFee;
}
public void setTotalFee(Integer totalFee) {
this.totalFee = totalFee;
}
public Integer getSettlementTotalFee() {
return settlementTotalFee;
}
public void setSettlementTotalFee(Integer settlementTotalFee) {
this.settlementTotalFee = settlementTotalFee;
}
public String getFeeType() {
return feeType;
}
public void setFeeType(String feeType) {
this.feeType = feeType;
}
public Integer getCashFee() {
return cashFee;
}
public void setCashFee(Integer cashFee) {
this.cashFee = cashFee;
}
public String getCashFeeType() {
return cashFeeType;
}
public void setCashFeeType(String cashFeeType) {
this.cashFeeType = cashFeeType;
}
public Integer getCouponFee() {
return couponFee;
}
public void setCouponFee(Integer couponFee) {
this.couponFee = couponFee;
}
public String getTransactionId() {
return transactionId;
}
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
public String getOutTradeNo() {
return outTradeNo;
}
public void setOutTradeNo(String outTradeNo) {
this.outTradeNo = outTradeNo;
}
public String getAttach() {
return attach;
}
public void setAttach(String attach) {
this.attach = attach;
}
public String getTimeEnd() {
return timeEnd;
}
public void setTimeEnd(String timeEnd) {
this.timeEnd = timeEnd;
}
public String getSubOpenid() {
return this.subOpenid;
}
public void setSubOpenid(String subOpenid) {
this.subOpenid = subOpenid;
}
public String getSubIsSubscribe() {
return this.subIsSubscribe;
}
public void setSubIsSubscribe(String subIsSubscribe) {
this.subIsSubscribe = subIsSubscribe;
}
@Override
public Map toMap() {
Map resultMap = BeanUtils.xmlBean2Map(this);
if (this.getCouponCount() != null && this.getCouponCount() > 0) {
for (int i = 0; i < this.getCouponCount(); i++) {
WxPayOrderNotifyCoupon coupon = couponList.get(i);
resultMap.putAll(coupon.toMap(i));
}
}
return resultMap;
}
@Override
public String toString() {
return ToStringUtils.toSimpleString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy