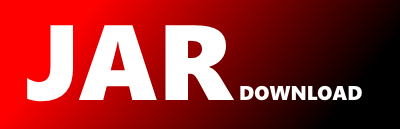
com.github.binarywang.wxpay.bean.result.WxPayRefundQueryResult Maven / Gradle / Ivy
package com.github.binarywang.wxpay.bean.result;
import com.google.common.collect.Lists;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import java.util.List;
/**
*
* Created by Binary Wang on 2016-11-24.
* @author binarywang(Binary Wang)
*
*/
@XStreamAlias("xml")
public class WxPayRefundQueryResult extends WxPayBaseResult {
/**
*
* 设备号
* device_info
* 否
* String(32)
* 013467007045764
* 终端设备号
*/
@XStreamAlias("device_info")
private String deviceInfo;
/**
*
* 微信订单号
* transaction_id
* 是
* String(32)
* 1217752501201407033233368018
* 微信订单号
*/
@XStreamAlias("transaction_id")
private String transactionId;
/**
*
* 商户订单号
* out_trade_no
* 是
* String(32)
* 1217752501201407033233368018
* 商户系统内部的订单号
*/
@XStreamAlias("out_trade_no")
private String outTradeNo;
/**
*
* 订单金额
* total_fee
* 是
* Int
* 100
* 订单总金额,单位为分,只能为整数,详见支付金额
*/
@XStreamAlias("total_fee")
private Integer totalFee;
/**
*
* 应结订单金额
* settlement_total_fee
* 否
* Int
* 100
* 应结订单金额=订单金额-非充值代金券金额,应结订单金额<=订单金额。
*/
@XStreamAlias("settlement_total_fee")
private Integer settlementTotalFee;
/**
*
* 货币种类
* fee_type
* 否
* String(8)
* CNY
* 订单金额货币类型,符合ISO 4217标准的三位字母代码,默认人民币:CNY,其他值列表详见货币类型
*/
@XStreamAlias("fee_type")
private String feeType;
/**
*
* 现金支付金额
* cash_fee
* 是
* Int
* 100
* 现金支付金额,单位为分,只能为整数,详见支付金额
*/
@XStreamAlias("cash_fee")
private Integer cashFee;
/**
*
* 退款笔数
* refund_count
* 是
* Int
* 1
* 退款记录数
*/
@XStreamAlias("refund_count")
private Integer refundCount;
private List refundRecords;
public String getDeviceInfo() {
return deviceInfo;
}
public void setDeviceInfo(String deviceInfo) {
this.deviceInfo = deviceInfo;
}
public String getTransactionId() {
return transactionId;
}
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
public String getOutTradeNo() {
return outTradeNo;
}
public void setOutTradeNo(String outTradeNo) {
this.outTradeNo = outTradeNo;
}
public Integer getTotalFee() {
return totalFee;
}
public void setTotalFee(Integer totalFee) {
this.totalFee = totalFee;
}
public Integer getSettlementTotalFee() {
return settlementTotalFee;
}
public void setSettlementTotalFee(Integer settlementTotalFee) {
this.settlementTotalFee = settlementTotalFee;
}
public String getFeeType() {
return feeType;
}
public void setFeeType(String feeType) {
this.feeType = feeType;
}
public Integer getCashFee() {
return cashFee;
}
public void setCashFee(Integer cashFee) {
this.cashFee = cashFee;
}
public Integer getRefundCount() {
return refundCount;
}
public void setRefundCount(Integer refundCount) {
this.refundCount = refundCount;
}
public List getRefundRecords() {
return refundRecords;
}
public void setRefundRecords(List refundRecords) {
this.refundRecords = refundRecords;
}
/**
* 组装生成退款记录属性的内容
*/
public void composeRefundRecords() {
if (this.refundCount != null && this.refundCount > 0) {
this.refundRecords = Lists.newArrayList();
for (int i = 0; i < this.refundCount; i++) {
RefundRecord refundRecord = new RefundRecord();
this.refundRecords.add(refundRecord);
refundRecord.setOutRefundNo(this.getXmlValue("xml/out_refund_no_" + i));
refundRecord.setRefundId(this.getXmlValue("xml/refund_id_" + i));
refundRecord.setRefundChannel(this.getXmlValue("xml/refund_channel_" + i));
refundRecord.setRefundFee(this.getXmlValueAsInt("xml/refund_fee_" + i));
refundRecord.setSettlementRefundFee(this.getXmlValueAsInt("xml/settlement_refund_fee_" + i));
refundRecord.setCouponType(this.getXmlValue("xml/coupon_type_" + i));
refundRecord.setCouponRefundFee(this.getXmlValueAsInt("xml/coupon_refund_fee_" + i));
refundRecord.setCouponRefundCount(this.getXmlValueAsInt("xml/coupon_refund_count_" + i));
refundRecord.setRefundStatus(this.getXmlValue("xml/refund_status_" + i));
refundRecord.setRefundRecvAccout(this.getXmlValue("xml/refund_recv_accout_" + i));
if (refundRecord.getCouponRefundCount() == null || refundRecord.getCouponRefundCount() == 0) {
continue;
}
List coupons = Lists.newArrayList();
for (int j = 0; j < refundRecord.getCouponRefundCount(); j++) {
coupons.add(
new RefundRecord.RefundCoupon(
this.getXmlValue("xml/coupon_refund_id_" + i + "_" + j),
this.getXmlValueAsInt("xml/coupon_refund_fee_" + i + "_" + j)
)
);
}
}
}
}
public static class RefundRecord {
/**
*
* 商户退款单号
* out_refund_no_$n
* 是
* String(32)
* 1217752501201407033233368018
* 商户退款单号
*
*/
@XStreamAlias("out_refund_no")
private String outRefundNo;
/**
*
* 微信退款单号
* refund_id_$n
* 是
* String(28)
* 1217752501201407033233368018
* 微信退款单号
*
*/
@XStreamAlias("refund_id")
private String refundId;
/**
*
* 退款渠道
* refund_channel_$n
* 否
* String(16)
* ORIGINAL
* ORIGINAL—原路退款 BALANCE—退回到余额
*
*/
@XStreamAlias("refund_channel")
private String refundChannel;
/**
*
* 申请退款金额
* refund_fee_$n
* 是
* Int
* 100
* 退款总金额,单位为分,可以做部分退款
*
*/
@XStreamAlias("refund_fee")
private Integer refundFee;
/**
*
* 退款金额
* settlement_refund_fee_$n
* 否
* Int
* 100
* 退款金额=申请退款金额-非充值代金券退款金额,退款金额<=申请退款金额
*
*/
@XStreamAlias("settlement_refund_fee")
private Integer settlementRefundFee;
/**
*
* 退款资金来源
* refund_account
* 否
* String(30)
* REFUND_SOURCE_RECHARGE_FUNDS
* REFUND_SOURCE_RECHARGE_FUNDS---可用余额退款/基本账户, REFUND_SOURCE_UNSETTLED_FUNDS---未结算资金退款
*
*/
@XStreamAlias("refund_account")
private String refundAccount;
/**
*
* 代金券类型
* coupon_type_$n
* 否
* Int
* CASH
* CASH--充值代金券 , NO_CASH---非充值代金券。订单使用代金券时有返回(取值:CASH、NO_CASH)。$n为下标,从0开始编号,举例:coupon_type_$0
*
*/
@XStreamAlias("coupon_type")
private String couponType;
/**
*
* 代金券退款金额
* coupon_refund_fee_$n
* 否
* Int
* 100
* 代金券退款金额<=退款金额,退款金额-代金券或立减优惠退款金额为现金,说明详见代金券或立减优惠
*
*/
@XStreamAlias("coupon_refund_fee")
private Integer couponRefundFee;
/**
*
* 退款代金券使用数量
* coupon_refund_count_$n
* 否
* Int
* 1
* 退款代金券使用数量 ,$n为下标,从0开始编号
*
*/
@XStreamAlias("coupon_refund_count")
private Integer couponRefundCount;
private List refundCoupons;
/**
*
* 退款状态
* refund_status_$n
* 是
* String(16)
* SUCCESS
* 退款状态:
* SUCCESS—退款成功,
* FAIL—退款失败,
* PROCESSING—退款处理中,
* CHANGE—转入代发,
* 退款到银行发现用户的卡作废或者冻结了,导致原路退款银行卡失败,资金回流到商户的现金帐号,需要商户人工干预,通过线下或者财付通转账的方式进行退款。
*
*/
@XStreamAlias("refund_status")
private String refundStatus;
/**
*
* 退款入账账户
* refund_recv_accout_$n
* 是
* String(64)
* 招商银行信用卡0403
* 取当前退款单的退款入账方,1)退回银行卡:{银行名称}{卡类型}{卡尾号},2)退回支付用户零钱:支付用户零钱
*
*/
@XStreamAlias("refund_recv_accout")
private String refundRecvAccout;
public String getOutRefundNo() {
return outRefundNo;
}
public void setOutRefundNo(String outRefundNo) {
this.outRefundNo = outRefundNo;
}
public String getRefundId() {
return refundId;
}
public void setRefundId(String refundId) {
this.refundId = refundId;
}
public String getRefundChannel() {
return refundChannel;
}
public void setRefundChannel(String refundChannel) {
this.refundChannel = refundChannel;
}
public Integer getRefundFee() {
return refundFee;
}
public void setRefundFee(Integer refundFee) {
this.refundFee = refundFee;
}
public Integer getSettlementRefundFee() {
return settlementRefundFee;
}
public void setSettlementRefundFee(Integer settlementRefundFee) {
this.settlementRefundFee = settlementRefundFee;
}
public String getRefundAccount() {
return refundAccount;
}
public void setRefundAccount(String refundAccount) {
this.refundAccount = refundAccount;
}
public String getCouponType() {
return couponType;
}
public void setCouponType(String couponType) {
this.couponType = couponType;
}
public Integer getCouponRefundFee() {
return couponRefundFee;
}
public void setCouponRefundFee(Integer couponRefundFee) {
this.couponRefundFee = couponRefundFee;
}
public Integer getCouponRefundCount() {
return couponRefundCount;
}
public void setCouponRefundCount(Integer couponRefundCount) {
this.couponRefundCount = couponRefundCount;
}
public List getRefundCoupons() {
return refundCoupons;
}
public void setRefundCoupons(List refundCoupons) {
this.refundCoupons = refundCoupons;
}
public String getRefundStatus() {
return refundStatus;
}
public void setRefundStatus(String refundStatus) {
this.refundStatus = refundStatus;
}
public String getRefundRecvAccout() {
return refundRecvAccout;
}
public void setRefundRecvAccout(String refundRecvAccout) {
this.refundRecvAccout = refundRecvAccout;
}
public static class RefundCoupon {
/**
*
* 退款代金券批次ID
* coupon_refund_batch_id_$n_$m
* 否
* String(20)
* 100
* 退款代金券批次ID ,$n为下标,$m为下标,从0开始编号
*
*
* @deprecated 貌似是被去掉了,但不知是何时!
*/
@XStreamAlias("coupon_refund_batch_id")
private String couponRefundBatchId;
/**
*
* 退款代金券ID
* coupon_refund_id_$n_$m
* 否
* String(20)
* 10000
* 退款代金券ID, $n为下标,$m为下标,从0开始编号
*
*/
@XStreamAlias("coupon_refund_id")
private String couponRefundId;
/**
*
* 单个退款代金券支付金额
* coupon_refund_fee_$n_$m
* 否
* Int
* 100
* 单个退款代金券支付金额, $n为下标,$m为下标,从0开始编号
*
*/
@XStreamAlias("coupon_refund_fee")
private Integer couponRefundFee;
public RefundCoupon(String couponRefundId, Integer couponRefundFee) {
this.couponRefundId = couponRefundId;
this.couponRefundFee = couponRefundFee;
}
@Deprecated
public RefundCoupon(String couponRefundBatchId, String couponRefundId, Integer couponRefundFee) {
this.couponRefundBatchId = couponRefundBatchId;
this.couponRefundId = couponRefundId;
this.couponRefundFee = couponRefundFee;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy