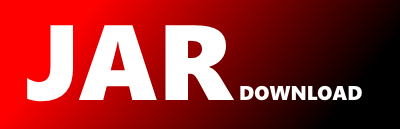
org.n3r.eql.util.HostAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eql Show documentation
Show all versions of eql Show documentation
a simple wrapper framework for jdbc to seperate sql and java code
package org.n3r.eql.util;
import java.io.Closeable;
import java.io.InputStream;
import java.net.*;
import java.util.Enumeration;
import java.util.Scanner;
public class HostAddress {
private static String ip;
private static String host;
static {
StringBuilder ips = new StringBuilder();
try {
Enumeration interfaces = NetworkInterface.getNetworkInterfaces();
while (interfaces.hasMoreElements()) {
NetworkInterface ni = interfaces.nextElement();
if (ni.isLoopback()) continue;
Enumeration inetAddresses = ni.getInetAddresses();
while (inetAddresses.hasMoreElements()) {
InetAddress inetAddress = inetAddresses.nextElement();
if (inetAddress instanceof Inet4Address) {
ips.append(inetAddress.getHostAddress()).append(",");
}
}
}
} catch (SocketException e) {
// ignore
}
if (ips.length() == 0) {
try {
InetAddress localHost = InetAddress.getLocalHost();
ip = localHost.getHostAddress();
} catch (UnknownHostException e) {
// ignore
}
} else {
ip = ips.deleteCharAt(ips.length() - 1).toString();
}
host = hostname();
}
public static String getHost() {
return host;
}
public static String getIp() {
return ip;
}
public static String hostname() {
Process proc = null;
InputStream stream = null;
Scanner s = null;
try {
proc = Runtime.getRuntime().exec("hostname");
stream = proc.getInputStream();
s = new Scanner(stream).useDelimiter("\\A");
return s.hasNext() ? s.next().trim() : "";
} catch (Exception e) {
return "unknown";
} finally {
closeQuietly(stream);
closeQuietly(s);
if (proc != null) proc.destroy();
if (s != null) s.close();
}
}
public static void closeQuietly(Closeable stream) {
if (stream != null) try {
stream.close();
} catch (Exception e) {
// ignore
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy