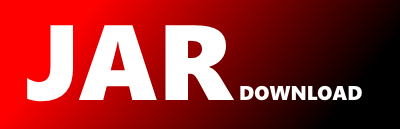
com.ibatis.common.jdbc.logging.ResultSetLogProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ibatis-enhanced Show documentation
Show all versions of ibatis-enhanced Show documentation
some enhanced features added for ibatis.
package com.ibatis.common.jdbc.logging;
import com.github.bingoohuang.ibatis.BlackcatUtils;
import com.ibatis.common.beans.ClassInfo;
import com.ibatis.common.logging.Log;
import com.ibatis.common.logging.LogFactory;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.sql.ResultSet;
/**
* ResultSet proxy to add logging
*/
public class ResultSetLogProxy extends BaseLogProxy implements InvocationHandler {
private static final Log log = LogFactory.getLog(ResultSet.class);
boolean first = true;
private ResultSet rs;
private ResultSetLogProxy(ResultSet rs) {
super();
this.rs = rs;
if (log.isDebugEnabled()) {
log.debug("{rset-" + id + "} ResultSet");
}
}
public Object invoke(Object proxy, Method method, Object[] params) throws Throwable {
try {
Object o = method.invoke(rs, params);
if (GET_METHODS.contains(method.getName())) {
if (params[0] instanceof String) {
setColumn(params[0], rs.wasNull() ? null : o);
}
} else if ("next".equals(method.getName())
|| "close".equals(method.getName())) {
String s = getValueString();
if (!"[]".equals(s)) {
if (first) {
first = false;
String columnString = getColumnString();
if (BlackcatUtils.HasBlackcat) {
BlackcatUtils.log("SQL.Header", columnString);
BlackcatUtils.log("SQL.Result", s);
}
if (log.isDebugEnabled()) {
log.debug("{rset-" + id + "} Header: " + columnString);
}
}
BlackcatUtils.log("SQL.Result", s);
if (log.isDebugEnabled()) {
log.debug("{rset-" + id + "} Result: " + s);
}
}
clearColumnInfo();
}
return o;
} catch (Throwable t) {
throw ClassInfo.unwrapThrowable(t);
}
}
/**
* Creates a logging version of a ResultSet
*
* @param rs - the ResultSet to proxy
* @return - the ResultSet with logging
*/
public static ResultSet newInstance(ResultSet rs) {
InvocationHandler handler = new ResultSetLogProxy(rs);
ClassLoader cl = ResultSet.class.getClassLoader();
return (ResultSet) Proxy.newProxyInstance(
cl, new Class[]{ResultSet.class}, handler);
}
/**
* Get the wrapped result set
*
* @return the resultSet
*/
public ResultSet getRs() {
return rs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy