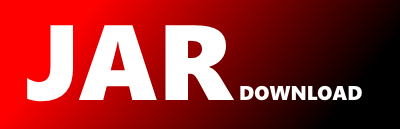
com.github.bingoohuang.utils.tuple.Tuple2 Maven / Gradle / Ivy
package com.github.bingoohuang.utils.tuple;
import lombok.*;
import java.util.Arrays;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
/**
* A tuple that holds two non-null values.
*
* @param The type of the first nullable value held by this tuple
* @param The type of the second nullable value held by this tuple
*/
@Getter @Setter @EqualsAndHashCode(callSuper = true) @AllArgsConstructor @NoArgsConstructor
public class Tuple2 extends Tuple1 {
T2 t2;
public Tuple2(T1 t1, T2 t2) {
super(t1);
this.t2 = t2;
}
/**
* Get the object at the given index.
*
* @param index The index of the object to retrieve. Starts at 0.
* @return The object or {@literal null} if out of bounds.
*/
public Object get(int index) {
switch (index) {
case 1:
return t2;
default:
return super.get(index);
}
}
/**
* Turn this {@literal Tuples} into a plain Object list.
*
* @return A new Object list.
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy