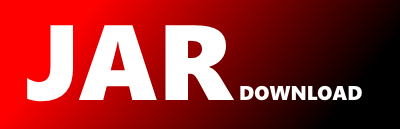
com.github.bingoohuang.voucherno.MessageDigestUtils Maven / Gradle / Ivy
package com.github.bingoohuang.voucherno;
import lombok.SneakyThrows;
import java.nio.charset.Charset;
import java.security.MessageDigest;
/**
* This program refers to the java-bloomfilter,you can get its details form https://github.com/MagnusS/Java-BloomFilter.
* You have any questions about this program please put issues on github to
* https://github.com/wxisme/bloomfilter
*/
public abstract class MessageDigestUtils {
private static final String MD5 = "MD5";//SHA1,SHA256
public static final Charset UTF8 = Charset.forName("UTF-8"); // encoding used for storing hash values as strings
private static final MessageDigest messageDigest = createMd5();
@SneakyThrows
public static MessageDigest createMd5() {
return MessageDigest.getInstance(MD5);
}
/**
* Generates digests based on the contents of an array of bytes and splits the result into 4-byte int's and store them in an array. The
* digest function is called until the required number of int's are produced. For each call to digest a salt
* is prepended to the data. The salt is increased by 1 for each call.
*
* @param data specifies input data.
* @param hashes number of hashes/int's to produce.
* @return array of int-sized hashes
*/
public static int[] createHashes(byte[] data, int hashes) {
int[] result = new int[hashes];
int k = 0;
byte salt = 0;
while (k < hashes) {
byte[] digest;
synchronized (messageDigest) {
messageDigest.update(salt);
salt++;
digest = messageDigest.digest(data);
}
for (int i = 0; i < digest.length / 4 && k < hashes; i++) {
int h = 0;
for (int j = (i * 4); j < (i * 4) + 4; j++) {
h <<= 8;
h |= ((int) digest[j]) & 0xFF;
}
result[k] = h;
k++;
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy