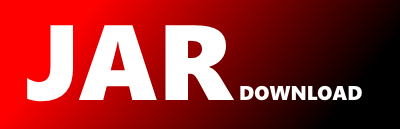
com.github.bjoernpetersen.jmusicbot.playback.Queue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of musicbot Show documentation
Show all versions of musicbot Show documentation
Core library of MusicBot, which plays music from various providers.
package com.github.bjoernpetersen.jmusicbot.playback;
import com.github.bjoernpetersen.jmusicbot.Song;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
import javax.annotation.Nonnull;
public final class Queue {
@Nonnull
private final LinkedList queue;
@Nonnull
private final Set listeners;
Queue() {
this.queue = new LinkedList<>();
this.listeners = new HashSet<>();
}
public void append(@Nonnull QueueEntry entry) {
Objects.requireNonNull(entry);
if (!queue.contains(entry)) {
queue.add(entry);
notifyListeners(listener -> listener.onAdd(entry));
}
}
public void remove(@Nonnull QueueEntry entry) {
Objects.requireNonNull(entry);
boolean removed = queue.remove(entry);
if (removed) {
notifyListeners(listener -> listener.onRemove(entry));
}
}
@Nonnull
public Optional pop() {
if (queue.isEmpty()) {
return Optional.empty();
} else {
QueueEntry entry = queue.pop();
notifyListeners(listener -> listener.onRemove(entry));
return Optional.of(entry);
}
}
public boolean isEmpty() {
return queue.isEmpty();
}
public void clear() {
queue.clear();
}
private Song get(int index) {
return queue.get(index).getSong();
}
public List toList() {
return Collections.unmodifiableList(queue);
}
/**
* Moves the specified QueueEntry to the specified index in the queue.
*
* - If the QueueEntry is not in the queue, this method does nothing.
- If the index
* is greater than the size of the queue, the entry is moved to the end of the queue.
*
* @param queueEntry a QueueEntry
* @param index a 0-based index
* @throws IllegalArgumentException if the index is smaller than 0
*/
public void move(QueueEntry queueEntry, int index) {
if (index < 0) {
throw new IllegalArgumentException("Index below 0");
}
int oldIndex = queue.indexOf(queueEntry);
if (oldIndex > -1) {
int newIndex = Math.min(queue.size() - 1, index);
if (oldIndex != newIndex) {
if (queue.remove(queueEntry)) {
queue.add(newIndex, queueEntry);
listeners.forEach(l -> l.onMove(queueEntry, oldIndex, newIndex));
}
}
}
}
public void addListener(@Nonnull QueueChangeListener listener) {
listeners.add(Objects.requireNonNull(listener));
}
public void removeListener(@Nonnull QueueChangeListener listener) {
listeners.remove(Objects.requireNonNull(listener));
}
private void notifyListeners(@Nonnull Consumer notifier) {
for (QueueChangeListener listener : listeners) {
notifier.accept(listener);
}
}
}