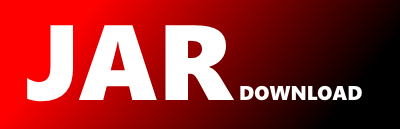
com.github.blackshadowwalker.spring.distributelock.interceptor.LockExpressionEvaluator Maven / Gradle / Ivy
package com.github.blackshadowwalker.spring.distributelock.interceptor;
import com.github.blackshadowwalker.spring.distributelock.Lock;
import org.springframework.aop.support.AopUtils;
import org.springframework.context.expression.AnnotatedElementKey;
import org.springframework.context.expression.CachedExpressionEvaluator;
import org.springframework.context.expression.MethodBasedEvaluationContext;
import org.springframework.core.DefaultParameterNameDiscoverer;
import org.springframework.core.ParameterNameDiscoverer;
import org.springframework.expression.EvaluationContext;
import org.springframework.expression.Expression;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Created by ASUS on 2016/8/16.
*/
public class LockExpressionEvaluator extends CachedExpressionEvaluator {
private final ParameterNameDiscoverer paramNameDiscoverer = new DefaultParameterNameDiscoverer();
private final Map lockCache = new ConcurrentHashMap(64);
private final Map targetMethodCache = new ConcurrentHashMap(64);
/**
* Create an {@link EvaluationContext}.
*
* @param locks
* the current locks
* @param method
* the method
* @param args
* the method arguments
* @param target
* the target object
* @param targetClass
* the target class
* @return the evaluation context
*/
public EvaluationContext createEvaluationContext(Collection extends Lock> locks, Object target, Class> targetClass, Method method,
Object[] args) {
LockExpressionRootObject rootObject = new LockExpressionRootObject(locks, method, args, target, targetClass);
Method targetMethod = getTargetMethod(targetClass, method);
MethodBasedEvaluationContext evaluationContext = new MethodBasedEvaluationContext(rootObject, targetMethod, args,
this.paramNameDiscoverer);
return evaluationContext;
}
public Object key(String keyExpression, AnnotatedElementKey methodKey, EvaluationContext evalContext) {
return getExpression(this.lockCache, methodKey, keyExpression).getValue(evalContext);
}
private Method getTargetMethod(Class> targetClass, Method method) {
AnnotatedElementKey methodKey = new AnnotatedElementKey(method, targetClass);
Method targetMethod = this.targetMethodCache.get(methodKey);
if (targetMethod == null) {
targetMethod = AopUtils.getMostSpecificMethod(method, targetClass);
if (targetMethod == null) {
targetMethod = method;
}
this.targetMethodCache.put(methodKey, targetMethod);
}
return targetMethod;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy