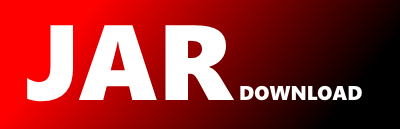
com.bld.commons.classes.model.ModelClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of class-generator Show documentation
Show all versions of class-generator Show documentation
Demo project for Spring Boot
The newest version!
/**
* @author Francesco Baldi
* @mail [email protected]
* @class bld.commons.classes.model.ModelClass.java
*/
package com.bld.commons.classes.model;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import com.bld.commons.classes.attributes.ClassType;
import com.bld.commons.classes.generator.utils.ClassGeneratorUtils;
import com.fasterxml.jackson.annotation.JsonProperty;
import jakarta.validation.Valid;
import jakarta.validation.constraints.NotNull;
/**
* The Class ModelClass.
*/
public class ModelClass implements ModelComponentClass {
/** The name. */
@NotNull(message = "The \"name\" field can not be null to define the \"class\" entity")
@JsonProperty("class")
private String name;
/** The type. */
@NotNull
private ClassType type;
/** The package name. */
@JsonProperty("package")
@NotNull
private String packageName;
/** The extends class. */
@JsonProperty("extends")
@Valid
private Set extendsClass;
/** The implements class. */
@JsonProperty("implements")
@Valid
private Set implementsClass;
/** The annotations. */
@Valid
private Set annotations;
/** The fields. */
@Valid
private LinkedHashSet fields;
/** The imports. */
private Set imports;
/** The generic types. */
@JsonProperty("generic-types")
private List genericTypes;
/** The lombok. */
private boolean lombok;
/** The abstract class. */
@JsonProperty("abstract")
private boolean abstractClass;
/** The methods. */
@Valid
private LinkedHashSet methods;
/** The costructors. */
private LinkedHashSet constructors;
/** The enum values. */
private LinkedHashSet enumValues;
/**
* Instantiates a new model class.
*/
public ModelClass() {
super();
init();
}
/**
* Inits the.
*/
private void init() {
this.annotations = new HashSet<>();
this.fields = new LinkedHashSet<>();
this.imports = new HashSet<>();
this.genericTypes = new ArrayList<>();
this.extendsClass = new HashSet<>();
this.implementsClass = new HashSet<>();
this.methods = new LinkedHashSet<>();
this.lombok = false;
this.abstractClass = false;
this.type = ClassType.CLASS;
this.constructors = new LinkedHashSet<>();
this.enumValues = new LinkedHashSet<>();
}
/**
* Instantiates a new model class.
*
* @param name the name
* @param type the type
* @param packageName the package name
*/
public ModelClass(String name, ClassType type, String packageName) {
super();
this.name = name;
this.type = type;
this.packageName = packageName;
init();
}
public void addInterface(ModelSuperClass... modelSuperClasses) {
ClassGeneratorUtils.addElements(this.implementsClass, modelSuperClasses);
}
public void addExtendsClass(ModelSuperClass... modelSuperClasses) {
ClassGeneratorUtils.addElements(this.extendsClass, modelSuperClasses);
}
public void addAnnotations(ModelAnnotation...annotations) {
ClassGeneratorUtils.addElements(this.annotations, annotations);
}
public void addFields(ModelField...fields) {
ClassGeneratorUtils.addElements(this.fields, fields);
}
/**
* Gets the name.
*
* @return the name
*/
public String getName() {
return name;
}
/**
* Sets the name.
*
* @param name the new name
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the package name.
*
* @return the package name
*/
public String getPackageName() {
return packageName;
}
/**
* Sets the package name.
*
* @param packageName the new package name
*/
public void setPackageName(String packageName) {
this.packageName = packageName;
}
/**
* Gets the annotations.
*
* @return the annotations
*/
public Set getAnnotations() {
return annotations;
}
/**
* Sets the annotations.
*
* @param annotations the new annotations
*/
public void setAnnotations(Set annotations) {
this.annotations = annotations;
}
/**
* Gets the fields.
*
* @return the fields
*/
public LinkedHashSet getFields() {
return fields;
}
/**
* Sets the fields.
*
* @param fields the new fields
*/
public void setFields(LinkedHashSet fields) {
this.fields = fields;
}
/**
* Gets the imports.
*
* @return the imports
*/
public Set getImports() {
return imports;
}
/**
* Sets the imports.
*
* @param imports the new imports
*/
public void setImports(Set imports) {
this.imports = imports;
}
/**
* Gets the generic types.
*
* @return the generic types
*/
public List getGenericTypes() {
return genericTypes;
}
/**
* Sets the generic types.
*
* @param genericType the new generic types
*/
public void setGenericTypes(List genericType) {
this.genericTypes = genericType;
}
/**
* Gets the extends class.
*
* @return the extends class
*/
public Set getExtendsClass() {
return extendsClass;
}
/**
* Sets the extends class.
*
* @param extendsClass the new extends class
*/
public void setExtendsClass(Set extendsClass) {
this.extendsClass = extendsClass;
}
/**
* Gets the implements class.
*
* @return the implements class
*/
public Set getImplementsClass() {
return implementsClass;
}
/**
* Sets the implements class.
*
* @param implementsClass the new implements class
*/
public void setImplementsClass(Set implementsClass) {
this.implementsClass = implementsClass;
}
/**
* Checks if is lombok.
*
* @return true, if is lombok
*/
public boolean isLombok() {
return lombok;
}
/**
* Sets the lombok.
*
* @param lombok the new lombok
*/
public void setLombok(boolean lombok) {
this.lombok = lombok;
}
/**
* Gets the type.
*
* @return the type
*/
public ClassType getType() {
return type;
}
/**
* Sets the type.
*
* @param type the new type
*/
public void setType(ClassType type) {
this.type = type;
}
/**
* Checks if is abstract class.
*
* @return true, if is abstract class
*/
public boolean isAbstractClass() {
return abstractClass;
}
/**
* Sets the abstract class.
*
* @param abstractClass the new abstract class
*/
public void setAbstractClass(boolean abstractClass) {
this.abstractClass = abstractClass;
}
/**
* Gets the methods.
*
* @return the methods
*/
public LinkedHashSet getMethods() {
return methods;
}
/**
* Sets the methods.
*
* @param methods the new methods
*/
public void setMethods(LinkedHashSet methods) {
this.methods = methods;
}
/**
* Gets the costructors.
*
* @return the costructors
*/
public LinkedHashSet getConstructors() {
return constructors;
}
/**
* Sets the costructors.
*
* @param constructors the new costructors
*/
public void setConstructors(LinkedHashSet constructors) {
this.constructors = constructors;
}
/**
* Gets the enum values.
*
* @return the enum values
*/
public LinkedHashSet getEnumValues() {
return enumValues;
}
/**
* Sets the enum values.
*
* @param enumValues the new enum values
*/
public void setEnumValues(LinkedHashSet enumValues) {
this.enumValues = enumValues;
}
public void addImport(String imp) {
if(StringUtils.isNotBlank(imp))
this.imports.add(imp);
}
/**
* To string.
*
* @return the string
*/
@Override
public String toString() {
String genericType = "";
for (ModelGenericType item : genericTypes)
genericType += "," + item.toString();
if (StringUtils.isNotEmpty(genericType))
genericType = "<" + genericType.substring(1) + ">";
String extend = "";
for (ModelSuperClass item : this.extendsClass)
extend += "," + item.toString();
if (StringUtils.isNotEmpty(extend))
extend = " extends " + extend.substring(1);
String implement = "";
for (ModelSuperClass item : this.implementsClass)
implement += "," + item.toString();
if (StringUtils.isNotEmpty(implement))
implement = " implements " + implement.substring(1);
String abstractClass = "";
if (this.abstractClass)
abstractClass = "abstract";
return "public " + abstractClass + " " + this.type.name().toLowerCase() + " " + this.name + genericType + ""
+ extend + "" + implement;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy