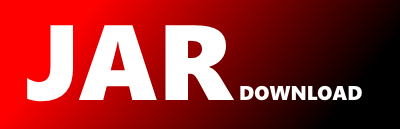
com.bld.commons.classes.model.ModelParameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of class-generator Show documentation
Show all versions of class-generator Show documentation
Demo project for Spring Boot
The newest version!
/**
* @author Francesco Baldi
* @mail [email protected]
* @class bld.commons.classes.model.ModelParameter.java
*/
package com.bld.commons.classes.model;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import com.bld.commons.classes.generator.annotation.FindImport;
import com.fasterxml.jackson.annotation.JsonProperty;
import jakarta.validation.Valid;
import jakarta.validation.constraints.NotNull;
/**
* The Class ModelParameter.
*/
public class ModelParameter implements ModelComponentClass {
/** The name. */
@JsonProperty("parameter")
@NotNull(message = "The \"parameter\" field can not be null to define the \"parameter\" entity")
private String name;
/** The type. */
@FindImport
@NotNull(message = "The \"type\" field can not be null to define the \"parameter\" entity")
private String type;
/** The parameter final. */
@JsonProperty("final")
private boolean parameterFinal;
/** The annotations. */
@Valid
private Set annotations;
/** The generic types. */
@JsonProperty("generic-types")
@Valid
private List genericTypes;
/**
* Instantiates a new model parameter.
*
* @param name the name
* @param type the type
*/
public ModelParameter(String name, String type) {
super();
this.name = name;
this.type = type;
this.annotations = new HashSet<>();
this.parameterFinal = false;
this.genericTypes = new ArrayList<>();
}
/**
* Instantiates a new model parameter.
*/
public ModelParameter() {
super();
this.annotations = new HashSet<>();
this.parameterFinal = false;
this.genericTypes = new ArrayList<>();
}
/**
* Gets the name.
*
* @return the name
*/
public String getName() {
return name;
}
/**
* Sets the name.
*
* @param name the new name
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the type.
*
* @return the type
*/
public String getType() {
return type;
}
/**
* Sets the type.
*
* @param type the new type
*/
public void setType(String type) {
this.type = type;
}
/**
* Gets the annotations.
*
* @return the annotations
*/
public Set getAnnotations() {
return annotations;
}
/**
* Sets the annotations.
*
* @param annotations the new annotations
*/
public void setAnnotations(Set annotations) {
this.annotations = annotations;
}
/**
* Checks if is parameter final.
*
* @return true, if is parameter final
*/
public boolean isParameterFinal() {
return parameterFinal;
}
/**
* Sets the parameter final.
*
* @param parameterFinal the new parameter final
*/
public void setParameterFinal(boolean parameterFinal) {
this.parameterFinal = parameterFinal;
}
/**
* Gets the generic types.
*
* @return the generic types
*/
public List getGenericTypes() {
return genericTypes;
}
/**
* Sets the generic types.
*
* @param genericTypes the new generic types
*/
public void setGenericTypes(List genericTypes) {
this.genericTypes = genericTypes;
}
/**
* To string.
*
* @return the string
*/
@Override
public String toString() {
String annotation = "";
for (ModelAnnotation item : this.annotations)
annotation += item.toString() + " ";
String genericType = "";
for (ModelGenericType item : genericTypes)
genericType += "," + (item.getName().equals("?") ? item.toString() : item.getName());
if (StringUtils.isNotEmpty(genericType))
genericType = "<" + genericType.substring(1) + ">";
return annotation + (this.parameterFinal ? " final" : "") + " " + type + genericType + " " + name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy