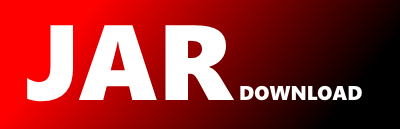
com.bld.commons.connection.utils.RestConnectionMapper Maven / Gradle / Ivy
/**
* @author Francesco Baldi
* @mail [email protected]
* @class com.bld.commons.connection.utils.RestConnectionMapper.java
*/
package com.bld.commons.connection.utils;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.commons.lang3.StringUtils;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.util.UriComponentsBuilder;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* The Class RestConnectionMapper.
*/
@SuppressWarnings({"unchecked"})
public class RestConnectionMapper {
/**
* From object to json.
*
* @param obj the obj
* @return the string
* @throws JsonProcessingException the json processing exception
*/
public static String fromObjectToJson(Object obj) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
String jsonObj = mapper.writerWithDefaultPrettyPrinter().writeValueAsString(obj);
return jsonObj;
}
/**
* From json to entity.
*
* @param the generic type
* @param json the json
* @param classObj the class obj
* @return the t
* @throws JsonParseException the json parse exception
* @throws JsonMappingException the json mapping exception
* @throws IOException Signals that an I/O exception has occurred.
*/
public static T fromJsonToEntity(String json, Class classObj)
throws JsonParseException, JsonMappingException, IOException {
ObjectMapper mapper = new ObjectMapper();
T obj = mapper.readValue(json, classObj);
return obj;
}
/**
* From object to map.
*
* @param obj the obj
* @return the map
* @throws JsonProcessingException the json processing exception
*/
public static Map fromObjectToMap(Object obj) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
Map map=new HashMap<>();
if(obj!=null) {
if(obj instanceof Map)
map=(Map)obj;
else
map=(Map) mapper.readValue(fromObjectToJson(obj), Map.class);
}
return map;
}
/**
* Builder query.
*
* @param builder the builder
* @param params the params
*/
public static void builderQuery(UriComponentsBuilder builder,Mapparams) {
builder.uriVariables(params);
for(Entryentry:params.entrySet())
builder.queryParam(entry.getKey(), entry.getValue());
}
/**
* Map to multi value map.
*
* @param params the params
* @return the multi value map
*/
public static MultiValueMap mapToMultiValueMap(Mapparams){
MultiValueMap multiValueMap=new LinkedMultiValueMap<>();
for(Entryentry:params.entrySet())
multiValueMap.add(entry.getKey(), entry.getValue());
return multiValueMap;
}
/**
* Builder query.
*
* @param builder the builder
* @param params the params
* @param nameObj the name obj
*/
public static void builderQuery(UriComponentsBuilder builder,Mapparams,String nameObj) {
builder.uriVariables(params);
for (Entry entry : params.entrySet()) {
if(entry.getValue() instanceof Map)
builderQuery(builder, (Map)entry.getValue(), entry.getKey()+".");
else if(entry.getValue() instanceof Collection) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy