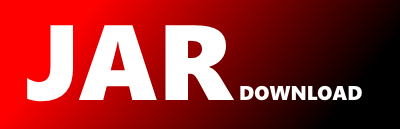
com.github.bloodshura.ignitium.cfg.csv.CsvReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-cfg Show documentation
Show all versions of ignitium-cfg Show documentation
A collection of configuration and serialization readers and writers, like JSON, internationalization (I18n), and CSV.
package com.github.bloodshura.ignitium.cfg.csv;
import com.github.bloodshura.ignitium.charset.Encoding;
import com.github.bloodshura.ignitium.charset.TextBuilder;
import com.github.bloodshura.ignitium.io.stream.TextReader;
import com.github.bloodshura.ignitium.resource.Resource;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.io.IOException;
import java.io.InputStream;
public class CsvReader extends CsvSpecification implements AutoCloseable {
private final TextReader parent;
public CsvReader(@Nonnull InputStream input) {
this(input, null);
}
public CsvReader(@Nonnull InputStream input, @Nullable Encoding encoding) {
this(new TextReader(input, encoding));
}
public CsvReader(@Nonnull Resource resource) throws IOException {
this(resource, null);
}
public CsvReader(@Nonnull Resource resource, @Nullable Encoding encoding) throws IOException {
this(new TextReader(resource, encoding));
}
public CsvReader(@Nonnull TextReader reader) {
this.parent = reader;
}
@Override
public void close() throws IOException {
parent.close();
}
@Nonnull
public Csv read() throws IOException {
Csv csv = new Csv();
CsvEntry entry;
while ((entry = readEntry()) != null) {
csv.add(entry);
}
return csv;
}
@Nullable
public CsvEntry readEntry() throws IOException {
CsvEntry entry = new CsvEntry();
TextBuilder builder = new TextBuilder();
boolean insideQuotes = false;
char[] buffer = new char[1];
while (parent.read(buffer) != -1) {
char ch = buffer[0];
if (ch == getSeparator() && !insideQuotes) {
if (!builder.isEmpty()) {
entry.add(builder.toStringAndClear());
}
} else if (ch == getQuote()) {
insideQuotes = !insideQuotes;
} else if ((ch == '\r' || ch == '\n') && !insideQuotes) {
if (!builder.isEmpty()) {
entry.add(builder.toStringAndClear());
return entry;
}
} else {
builder.append(ch);
}
}
if (!builder.isEmpty()) {
entry.add(builder.toStringAndClear());
}
return !entry.isEmpty() ? entry : null;
}
@Nonnull
public Csv readWithHeader() throws IOException {
CsvEntry header = readEntry();
Csv csv = new Csv(header);
CsvEntry entry;
while ((entry = readEntry()) != null) {
csv.add(entry);
}
return csv;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy