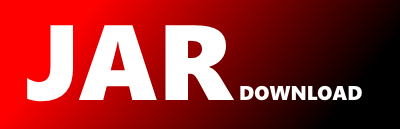
com.github.bloodshura.ignitium.cfg.json.JsonObject Maven / Gradle / Ivy
package com.github.bloodshura.ignitium.cfg.json;
import com.github.bloodshura.ignitium.cfg.AbstractTransformableMapping;
import com.github.bloodshura.ignitium.cfg.json.JsonParseException.ErrorType;
import com.github.bloodshura.ignitium.cfg.json.handling.JsonComposer;
import com.github.bloodshura.ignitium.cfg.json.handling.JsonParser;
import com.github.bloodshura.ignitium.collection.map.impl.XLinkedMap;
import com.github.bloodshura.ignitium.collection.tuple.Pair;
import com.github.bloodshura.ignitium.exception.UnexpectedException;
import com.github.bloodshura.ignitium.resource.InMemoryOutputResource;
import com.github.bloodshura.ignitium.resource.OutputResource;
import com.github.bloodshura.ignitium.resource.Resource;
import com.github.bloodshura.ignitium.resource.StringResource;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.io.IOException;
public final class JsonObject extends AbstractTransformableMapping implements Json {
public JsonObject() {
super(new XLinkedMap() {
@Override
public boolean canInsert(@Nonnull Pair object) {
return super.canInsert(object) && JsonType.isJson(object.getRight());
}
});
}
public JsonObject(@Nonnull CharSequence content) throws IOException, JsonParseException {
this(new StringResource(content));
}
public JsonObject(@Nonnull Resource resource) throws IOException, JsonParseException {
this();
load(resource);
}
public boolean contains(@Nonnull String key, @Nonnull JsonType type) {
return typeOf(key) == type;
}
@Nullable
public Object get(@Nonnull String key, @Nonnull JsonType type) {
return get(key, type, null);
}
@Nullable
public Object get(@Nonnull String key, @Nonnull JsonType type, @Nullable Object defaultValue) {
Object value = get(key);
if (value != null && JsonType.forValue(value) == type) {
return value;
}
return defaultValue;
}
@Nullable
public JsonArray getArray(@Nonnull String key) {
return getArray(key, null);
}
@Nullable
public JsonArray getArray(@Nonnull String key, @Nullable JsonArray defaultValue) {
return defaultGet(key, JsonArray.class, defaultValue);
}
@Nullable
public JsonObject getChild(@Nonnull String key) {
return getChild(key, null);
}
@Nullable
public JsonObject getChild(@Nonnull String key, @Nullable JsonObject defaultValue) {
return defaultGet(key, JsonObject.class, defaultValue);
}
public void load(@Nonnull JsonObject object) {
clear();
entries().addAll(object);
}
@Override
public void load(@Nonnull Resource resource) throws IOException, JsonParseException {
clear();
Object result = JsonParser.parse(resource);
if (result instanceof JsonObject) {
load((JsonObject) result);
} else if (result != null) {
throw new JsonParseException(ErrorType.UNEXPECTED_VALUE, 0, "Parsed resource has type " + result.getClass().getSimpleName() + "; expected " + getClass().getSimpleName());
} else {
throw new JsonParseException(ErrorType.UNEXPECTED_VALUE, 0, "Parsed resource is null; expected to be a JSON object");
}
}
@Override
public void save(@Nonnull OutputResource resource) throws IOException {
JsonComposer.compose(this, resource, true);
}
@Nonnull
@Override
public String toString() {
return toString(true);
}
@Nonnull
public String toString(boolean pretty) {
try {
InMemoryOutputResource resource = new InMemoryOutputResource();
JsonComposer.compose(this, resource, pretty);
return resource.getString();
} catch (IOException exception) { // Teorically should not happen
throw new UnexpectedException(exception);
}
}
@Nullable
public JsonType typeOf(@Nonnull String key) {
Object value = get(key);
return value != null ? JsonType.forValue(get(key)) : null;
}
public static boolean isJsonValue(@Nonnull Object value) {
return JsonType.forValue(value) != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy