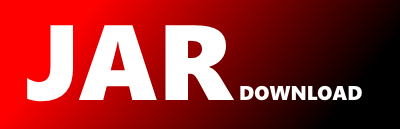
com.github.bloodshura.ignitium.cfg.json.mapping.CollectionInstantiator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-cfg Show documentation
Show all versions of ignitium-cfg Show documentation
A collection of configuration and serialization readers and writers, like JSON, internationalization (I18n), and CSV.
package com.github.bloodshura.ignitium.cfg.json.mapping;
import com.github.bloodshura.ignitium.collection.list.XList;
import com.github.bloodshura.ignitium.collection.list.impl.XArrayList;
import com.github.bloodshura.ignitium.collection.map.XMap;
import com.github.bloodshura.ignitium.collection.map.impl.XLinkedMap;
import com.github.bloodshura.ignitium.collection.set.XSet;
import com.github.bloodshura.ignitium.collection.set.impl.XArraySet;
import com.github.bloodshura.ignitium.collection.store.XStore;
import com.github.bloodshura.ignitium.collection.view.XBasicView;
import com.github.bloodshura.ignitium.collection.view.XView;
import javax.annotation.Nonnull;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
final class CollectionInstantiator {
@Nonnull
public static Constructor> getViewConstructor(@Nonnull Class> type) throws JsonMappingException {
try {
return type.getDeclaredConstructor(XStore.class);
} catch (NoSuchMethodException exception) {
try {
return type.getDeclaredConstructor(XList.class);
} catch (NoSuchMethodException exception2) {
try {
return type.getDeclaredConstructor(XArrayList.class);
} catch (NoSuchMethodException exception3) {
throw new JsonMappingException(type.getName() + " is a XView subclass but does not implement a constructor which parameter type is XStore, or XList, or XArrayList");
}
}
}
}
@Nonnull
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy