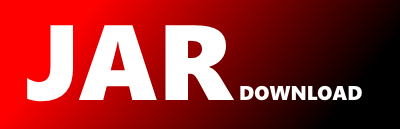
com.github.bloodshura.ignitium.cfg.json.mapping.JsonSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-cfg Show documentation
Show all versions of ignitium-cfg Show documentation
A collection of configuration and serialization readers and writers, like JSON, internationalization (I18n), and CSV.
package com.github.bloodshura.ignitium.cfg.json.mapping;
import com.github.bloodshura.ignitium.cfg.json.Json;
import com.github.bloodshura.ignitium.cfg.json.JsonArray;
import com.github.bloodshura.ignitium.cfg.json.JsonObject;
import com.github.bloodshura.ignitium.cfg.json.mapping.annotation.Mapped;
import com.github.bloodshura.ignitium.collection.list.XList;
import com.github.bloodshura.ignitium.collection.list.impl.XArrayList;
import com.github.bloodshura.ignitium.collection.map.XMap;
import com.github.bloodshura.ignitium.collection.tuple.Pair;
import com.github.bloodshura.ignitium.object.Base;
import javax.annotation.Nonnull;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Map;
import java.util.function.Predicate;
public class JsonSerializer {
@Nonnull
public static JsonObject serialize(@Nonnull JsonSerializable element) throws JsonMappingException {
JsonObject json = new JsonObject();
XList fields = new XArrayList<>();
Class> current = element.getClass();
Predicate fieldFilter = field -> !Modifier.isStatic(field.getModifiers()) && (!field.isAnnotationPresent(Mapped.class) || !field.getAnnotation(Mapped.class).excluded());
while (current != null && current != Base.class && current != Enum.class) {
fields.addAllIf(fieldFilter, current.getDeclaredFields());
current = current.getSuperclass();
}
for (Field field : fields) {
field.setAccessible(true);
try {
json.set(field.getName(), serializeElement(element.getClass(), field, field.get(element)));
} catch (IllegalAccessException exception) {
throw new JsonMappingException(exception);
} finally {
field.setAccessible(false);
}
}
if (element instanceof Enum>) {
String nameKey = json.contains("name") ? "enumName" : "name";
json.set(nameKey, ((Enum>) element).name().toLowerCase());
}
return json;
}
@Nonnull
public static JsonArray serializeAll(@Nonnull Iterable> elements) throws JsonMappingException {
JsonArray json = new JsonArray();
for (Object element : elements) {
if (element instanceof JsonSerializable) {
json.add(serialize((JsonSerializable) element));
}
}
return json;
}
private static Object serializeElement(Class> structureType, Field origin, Object element) throws JsonMappingException {
if (origin != null && origin.isAnnotationPresent(Mapped.class)) {
Mapped configuration = origin.getAnnotation(Mapped.class);
Class extends ValueMapper> mapperClass = configuration.serializer();
if (mapperClass != ValueMapper.class) {
ValueMapper mapper = ValueMapper.createMapper(mapperClass, structureType, origin.getType(), origin.getName());
element = mapper != null ? mapper.map(element) : element;
}
}
if (element instanceof JsonSerializable) {
return serialize((JsonSerializable) element);
}
if (element instanceof Json) {
return element;
}
if (element instanceof Map, ?>) {
JsonObject object = new JsonObject();
for (Map.Entry, ?> entry : ((Map, ?>) element).entrySet()) {
Object key = entry.getKey();
Object value = entry.getValue();
if (key != null) {
object.set(key.toString(), serializeElement(structureType, null, value));
}
}
return object;
}
if (element instanceof XMap, ?>) {
JsonObject object = new JsonObject();
for (Pair, ?> entry : (XMap, ?>) element) {
Object key = entry.getLeft();
Object value = entry.getRight();
if (key != null) {
object.set(key.toString(), serializeElement(structureType, null, value));
}
}
return object;
}
if (element instanceof Iterable>) {
JsonArray array = new JsonArray();
for (Object obj : (Iterable>) element) {
if (obj != null) {
array.add(serializeElement(structureType, null, obj));
}
}
return array;
}
if (element instanceof Enum>) {
return element.toString();
}
return element;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy