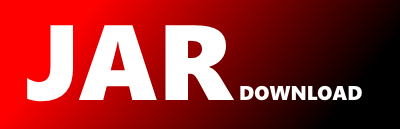
com.github.bloodshura.ignitium.cfg.json.mapping.Parameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-cfg Show documentation
Show all versions of ignitium-cfg Show documentation
A collection of configuration and serialization readers and writers, like JSON, internationalization (I18n), and CSV.
package com.github.bloodshura.ignitium.cfg.json.mapping;
import com.github.bloodshura.ignitium.object.AutoBase;
import com.github.bloodshura.ignitium.worker.UtilWorker;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
public final class Parameter extends AutoBase {
private final Class> expectedType;
private final boolean ignoresCase;
private final String name;
private final boolean optional;
private final Object origin;
private Object value;
private final Class extends ValueMapper> valueMapper;
public Parameter(@Nonnull Object origin, @Nonnull String name, @Nonnull Class> expectedType, boolean optional, boolean ignoresCase, @Nullable Class extends ValueMapper> valueMapper) {
this.expectedType = expectedType;
this.ignoresCase = ignoresCase;
this.name = name;
this.optional = optional;
this.origin = origin;
this.valueMapper = valueMapper;
}
public boolean accepts(@Nonnull Class> valueType) {
return getExpectedType().isAssignableFrom(valueType) || UtilWorker.primitiveClassMatch(valueType, getExpectedType()) || testImplicitNumericCoercion(valueType);
}
public boolean accepts(@Nullable Object value) {
return value == null ? isOptional() : accepts(value.getClass());
}
@Nonnull
public Class> getExpectedType() {
return expectedType;
}
@Nonnull
public String getName() {
return name;
}
@Nullable
public Object getOrigin() {
return origin;
}
@Nullable
public Object getValue() {
return value;
}
@Nullable
public Class extends ValueMapper> getValueMapper() {
return valueMapper;
}
public boolean ignoresCase() {
return ignoresCase;
}
public boolean isOptional() {
return optional;
}
public void setValue(@Nullable Object value) throws JsonMappingException {
if (value == null) {
this.value = null;
} else if (accepts(value)) {
this.value = value;
} else {
throw new JsonMappingException("Value is not accepted (expected type: " + getExpectedType().getSimpleName() + ", value type: " + value.getClass().getSimpleName() + ')');
}
}
private boolean testImplicitNumericCoercion(Class> valueType) {
Class> paramType = UtilWorker.fixPrimitiveClass(getExpectedType());
valueType = UtilWorker.fixPrimitiveClass(valueType);
return Number.class.isAssignableFrom(paramType) && Number.class.isAssignableFrom(valueType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy