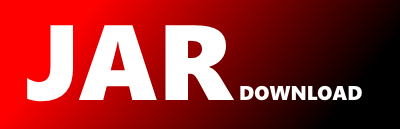
com.github.bloodshura.ignitium.cfg.json.mapping.ValueMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-cfg Show documentation
Show all versions of ignitium-cfg Show documentation
A collection of configuration and serialization readers and writers, like JSON, internationalization (I18n), and CSV.
package com.github.bloodshura.ignitium.cfg.json.mapping;
import com.github.bloodshura.ignitium.enumeration.Enumerations;
import com.github.bloodshura.ignitium.worker.ParseWorker;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
public interface ValueMapper {
@Nullable
default Object defaultValue() {
return null;
}
@Nullable
Object map(@Nullable Object value);
final class ByIdentifier implements ValueMapper {
private final Class extends Enum>> type;
public ByIdentifier(Class extends Enum>> type) {
this.type = type;
}
@Nullable
@Override
public Object map(@Nullable Object value) {
return value != null ? Enumerations.search(type, value.toString()) : null;
}
}
final class ByOrdinal implements ValueMapper {
private final Class extends Enum>> type;
public ByOrdinal(Class extends Enum>> type) {
this.type = type;
}
@Nullable
@Override
public Object map(@Nullable Object value) {
if (value == null) {
return null;
}
int index = ParseWorker.toInt(value.toString(), -1);
Enum>[] constants = type.getEnumConstants();
return index >= 0 && index < constants.length ? constants[index] : null;
}
}
@Nullable
static ValueMapper createMapper(@Nullable Class extends ValueMapper> mapperClass, @Nonnull Class> structureType, @Nonnull Class> type, @Nonnull String paramName) throws JsonMappingException {
if (type.isEnum()) {
try {
ValueMapper mapper;
if (mapperClass != null) {
try {
try {
Constructor extends ValueMapper> constructor = mapperClass.getDeclaredConstructor(Class.class);
try {
constructor.setAccessible(true);
mapper = constructor.newInstance(type);
} finally {
constructor.setAccessible(false);
}
} catch (NoSuchMethodException exception) {
Constructor extends ValueMapper> constructor = mapperClass.getDeclaredConstructor();
try {
constructor.setAccessible(true);
mapper = constructor.newInstance();
} finally {
constructor.setAccessible(false);
}
}
} catch (InvocationTargetException exception) {
throw new JsonMappingException("Could not instantiate the value mapper \"" + mapperClass.getName() + "\" of " + structureType.getSimpleName() + "::" + paramName, exception);
} catch (NoSuchMethodException exception) {
throw new JsonMappingException("Could not find suitable constructor for value mapper \"" + mapperClass.getName() + "\" of " + structureType.getSimpleName() + "::" + paramName);
}
} else {
mapper = new ValueMapper.ByIdentifier((Class extends Enum>>) type);
}
return mapper;
} catch (IllegalAccessException | InstantiationException exception) {
throw new JsonMappingException("Could not instantiate the value mapper \"" + mapperClass.getName() + "\" of " + structureType.getSimpleName() + "::" + paramName);
}
} else if (mapperClass != null) {
try {
ValueMapper mapper;
try {
Constructor extends ValueMapper> constructor = mapperClass.getDeclaredConstructor();
try {
constructor.setAccessible(true);
mapper = constructor.newInstance();
} finally {
constructor.setAccessible(false);
}
} catch (InvocationTargetException exception) {
throw new JsonMappingException("Could not instantiate the value mapper \"" + mapperClass.getName() + "\" of " + structureType.getSimpleName() + "::" + paramName, exception);
} catch (NoSuchMethodException exception) {
throw new JsonMappingException("Could not find suitable constructor for value mapper \"" + mapperClass.getName() + "\" of " + structureType.getSimpleName() + "::" + paramName);
}
return mapper;
} catch (IllegalAccessException | InstantiationException exception) {
throw new JsonMappingException("Could not instantiate the value mapper \"" + mapperClass.getName() + "\" of " + structureType.getSimpleName() + "::" + paramName);
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy