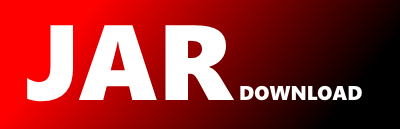
com.github.bloodshura.ignitium.cfg.props.serial.PropertySerializationAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-cfg Show documentation
Show all versions of ignitium-cfg Show documentation
A collection of configuration and serialization readers and writers, like JSON, internationalization (I18n), and CSV.
package com.github.bloodshura.ignitium.cfg.props.serial;
import com.github.bloodshura.ignitium.object.Base;
import com.github.bloodshura.ignitium.object.Mutable;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
@Mutable
public class PropertySerializationAttributes extends Base {
private char charIndexer;
private char commenter;
private char listCloser;
private char listOpener;
private char listSeparator;
private char separator;
private String separatorSuffix;
private char stringIndexer;
public PropertySerializationAttributes() {
setCharIndexer('\'');
setCommenter('#');
setListCloser(']');
setListOpener('[');
setListSeparator(',');
setSeparator(':');
setSeparatorSuffix(" ");
setStringIndexer('"');
}
public char getCharIndexer() {
return charIndexer;
}
public char getCommenter() {
return commenter;
}
public char getListCloser() {
return listCloser;
}
public char getListOpener() {
return listOpener;
}
public char getListSeparator() {
return listSeparator;
}
public char getSeparator() {
return separator;
}
@Nullable
public String getSeparatorSuffix() {
return separatorSuffix;
}
public char getStringIndexer() {
return stringIndexer;
}
public boolean hasSeparatorSuffix() {
return getSeparatorSuffix() != null;
}
public void setCharIndexer(char charIndexer) {
this.charIndexer = charIndexer;
}
public void setCommenter(char commenter) {
this.commenter = commenter;
}
public void setListCloser(char listCloser) {
this.listCloser = listCloser;
}
public void setListOpener(char listOpener) {
this.listOpener = listOpener;
}
public void setListSeparator(char listSeparator) {
this.listSeparator = listSeparator;
}
public void setSeparator(char separator) {
this.separator = separator;
}
public void setSeparatorSuffix(@Nullable String separatorSuffix) {
this.separatorSuffix = separatorSuffix;
}
public void setStringIndexer(char stringIndexer) {
this.stringIndexer = stringIndexer;
}
@Nonnull
@Override
protected Object[] stringValues() {
return new Object[] { getCharIndexer(), getCommenter(), getListCloser(), getListOpener(), getListSeparator(), getSeparator(), getSeparatorSuffix(), getStringIndexer() };
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy