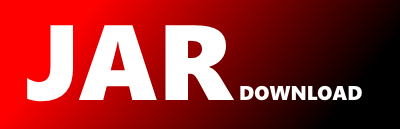
com.github.bloodshura.ignitium.ntv.mouse.impl.X11MouseHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-native Show documentation
Show all versions of ignitium-native Show documentation
An API for working with native system APIs, with a higher-level interface.
The newest version!
package com.github.bloodshura.ignitium.ntv.mouse.impl;
import com.github.bloodshura.ignitium.geometry.position.Position;
import com.github.bloodshura.ignitium.ntv.ErrorCodeNativeException;
import com.github.bloodshura.ignitium.ntv.NativeException;
import com.github.bloodshura.ignitium.ntv.mouse.AbstractMouseHandler;
import com.github.bloodshura.ignitium.worker.ArrayWorker;
import com.sun.jna.platform.unix.X11;
import com.sun.jna.platform.unix.X11.Display;
import com.sun.jna.platform.unix.X11.Window;
import com.sun.jna.platform.unix.X11.WindowByReference;
import com.sun.jna.ptr.IntByReference;
import javax.annotation.Nonnull;
// TODO Test (and improve) behaviour when on multi-screen devices...
public class X11MouseHandler extends AbstractMouseHandler {
private Display display;
private final IntByReference[] references;
public X11MouseHandler() throws NativeException {
this.display = X11.INSTANCE.XOpenDisplay(null);
this.references = new IntByReference[5];
if (display == null) {
throw new ErrorCodeNativeException("Could not open X11 display");
}
ArrayWorker.fill(references, IntByReference::new);
}
@Override
public void dispose() throws NativeException {
ensureNotDisposed();
int status = X11.INSTANCE.XCloseDisplay(display);
if (status != X11.Success) {
throw new ErrorCodeNativeException("Could not dispose X11 display", status);
}
this.display = null;
ArrayWorker.fill(references, () -> null);
}
@Nonnull
@Override
public Position getPosition() throws NativeException {
ensureNotDisposed();
int screen = X11.INSTANCE.XDefaultScreen(display);
Window window = X11.INSTANCE.XRootWindow(display, screen);
synchronized (references) {
if (X11.INSTANCE.XQueryPointer(display, window, new WindowByReference(), new WindowByReference(), references[0], references[1], references[2], references[3], references[4])) {
return new Position(references[0].getValue(), references[1].getValue());
}
}
throw new ErrorCodeNativeException("Could not query X11 pointer location");
}
private void ensureNotDisposed() throws NativeException {
if (display == null) {
throw new NativeException("Mouse handler already disposed");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy