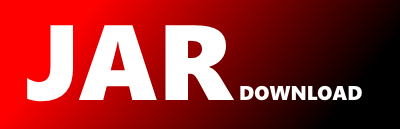
com.github.bloodshura.ignitium.ntv.sys.impl.Win32NativeSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ignitium-native Show documentation
Show all versions of ignitium-native Show documentation
An API for working with native system APIs, with a higher-level interface.
The newest version!
package com.github.bloodshura.ignitium.ntv.sys.impl;
import com.github.bloodshura.ignitium.ntv.ErrorCodeNativeException;
import com.github.bloodshura.ignitium.ntv.NativeException;
import com.github.bloodshura.ignitium.ntv.lib.Win32;
import com.github.bloodshura.ignitium.ntv.sys.NativeSystem;
import com.github.bloodshura.ignitium.util.XApi;
import com.sun.jna.platform.win32.Advapi32;
import com.sun.jna.platform.win32.Advapi32Util;
import com.sun.jna.platform.win32.Kernel32;
import com.sun.jna.platform.win32.WinNT.HANDLEByReference;
import com.sun.jna.platform.win32.WinNT.PSIDByReference;
import com.sun.jna.platform.win32.WinNT.TOKEN_INFORMATION_CLASS;
import com.sun.jna.platform.win32.WinNT.WELL_KNOWN_SID_TYPE;
import com.sun.jna.ptr.IntByReference;
import javax.annotation.Nonnull;
public class Win32NativeSystem implements NativeSystem {
@Override
public void beep() throws NativeException {
beep(0x3000, 500);
}
public void beep(int frequency, int duration) throws NativeException {
XApi.require(frequency >= 0x25 && frequency <= 0x7FFF, "Invalid frequency parameter: must be in range 0x25..0x7FFF");
XApi.require(duration >= 0, "Duration must be positive");
if (!Win32.Kernel.Beep(frequency, duration)) {
throw new ErrorCodeNativeException("Could not emit a beep");
}
}
@Override
public int getProcessId() throws NativeException {
return Kernel32.INSTANCE.GetCurrentProcessId();
}
@Override
public boolean isProcessPrivileged() throws NativeException {
HANDLEByReference token = new HANDLEByReference();
if (Advapi32.INSTANCE.OpenProcessToken(Kernel32.INSTANCE.GetCurrentProcess(), Kernel32.TOKEN_QUERY, token)) {
Win32.TOKEN_ELEVATED structure = new Win32.TOKEN_ELEVATED();
IntByReference unused = new IntByReference();
if (Advapi32.INSTANCE.GetTokenInformation(token.getValue(), TOKEN_INFORMATION_CLASS.TokenElevation, structure, structure.size(), unused)) {
try {
return structure.TokenIsElevated.intValue() != 0x0;
} finally {
Kernel32.INSTANCE.CloseHandle(token.getValue());
}
}
}
return false;
}
@Override
public boolean isUserAdmin() throws NativeException {
for (Advapi32Util.Account group : Advapi32Util.getCurrentUserGroups()) {
PSIDByReference sid = new PSIDByReference();
if (Advapi32.INSTANCE.ConvertStringSidToSid(group.sidString, sid)) {
if (Advapi32.INSTANCE.IsWellKnownSid(sid.getValue(), WELL_KNOWN_SID_TYPE.WinBuiltinAdministratorsSid)) {
return true;
}
}
}
return false;
}
public void messageBeep(@Nonnull MessageBeepType type) throws NativeException {
if (!Win32.Kernel.MessageBeep(type.value)) {
throw new ErrorCodeNativeException("Could not emit a message beep");
}
}
public static enum MessageBeepType {
ERROR(0x10),
INFORMATION(0x40),
OK(0x0),
QUESTION(0x20),
WARNING(0x30);
private final int value;
private MessageBeepType(int value) {
this.value = value;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy