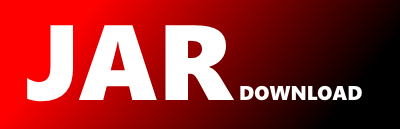
com.github.bloodshura.x.assets.AssetLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets;
import com.github.bloodshura.x.activity.logging.XLogger;
import com.github.bloodshura.x.assets.exception.AssetException;
import com.github.bloodshura.x.assets.font.Font;
import com.github.bloodshura.x.assets.font.truetype.TrueTypeFont;
import com.github.bloodshura.x.assets.image.Image;
import com.github.bloodshura.x.assets.image.ImageFormat;
import com.github.bloodshura.x.assets.image.ImageSource;
import com.github.bloodshura.x.assets.sound.Sound;
import com.github.bloodshura.x.assets.sound.SoundFormat;
import com.github.bloodshura.x.assets.sound.SoundSource;
import com.github.bloodshura.x.assets.util.ImageWorker;
import com.github.bloodshura.x.collection.list.XList;
import com.github.bloodshura.x.collection.list.impl.XArrayList;
import com.github.bloodshura.x.collection.util.Cache;
import com.github.bloodshura.x.collection.view.XBasicView;
import com.github.bloodshura.x.collection.view.XView;
import com.github.bloodshura.x.io.Url;
import com.github.bloodshura.x.io.file.File;
import com.github.bloodshura.x.loader.ResourceLoader;
import com.github.bloodshura.x.loader.ResourceNotFoundException;
import com.github.bloodshura.x.resource.Resource;
import com.github.bloodshura.x.worker.StringWorker;
import javax.annotation.Nonnull;
import javax.swing.Icon;
import javax.swing.filechooser.FileSystemView;
import java.awt.FontFormatException;
import java.awt.GraphicsEnvironment;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteBuffer;
public class AssetLoader extends Cache {
private XView localFonts;
private ResourceLoader resourceLoader;
public AssetLoader() {
this.resourceLoader = ResourceLoader.getDefault();
}
@Nonnull
public Image getImage(@Nonnull ByteBuffer input, int width, int height) throws AssetException {
return getImage(ImageSource.of(input, width, height));
}
@Nonnull
public Image getImage(@Nonnull File file) throws AssetException {
return getImage(ImageSource.of(file));
}
@Nonnull
public Image getImage(@Nonnull File file, @Nonnull ImageFormat format) throws AssetException {
return getImage(ImageSource.of(file, format));
}
@Nonnull
public Image getImage(@Nonnull ImageFormat format, @Nonnull byte[] input) throws AssetException {
return getImage(ImageSource.of(format, input));
}
@Nonnull
public Image getImage(@Nonnull ImageFormat format, @Nonnull InputStream input) throws AssetException {
return getImage(ImageSource.of(format, input));
}
@Nonnull
public Image getImage(@Nonnull ImageFormat format, @Nonnull java.awt.Image image) throws AssetException {
return getImage(ImageSource.of(format, image));
}
@Nonnull
public Image getImage(@Nonnull ImageFormat format, @Nonnull Resource input) throws AssetException {
return getImage(ImageSource.of(format, input));
}
@Nonnull
public Image getImage(@Nonnull ImageSource source) throws AssetException {
if (assertType(source.getName(), Image.class)) {
Image image = (Image) getCached(source.getName());
if (image.getFormat() == source.getFormat()) {
return image;
}
XLogger.internal("Image cached \"" + source.getName() + "\" but different format (source: " + source.getFormat() + ", cached: " + image.getFormat() + ')');
}
Image image = source.load();
insert(source.getName(), image);
return image;
}
@Nonnull
public Image getImage(@Nonnull int[] pixels, int width, int height) throws AssetException {
return getImage(ImageSource.of(pixels, width, height));
}
@Nonnull
public Image getImage(@Nonnull java.awt.Image image) throws AssetException {
return getImage(ImageSource.of(image));
}
@Nonnull
public Image getImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull byte[] input) throws AssetException {
return getImage(ImageSource.of(name, format, input));
}
@Nonnull
public Image getImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull InputStream input) throws AssetException {
return getImage(ImageSource.of(name, format, input));
}
@Nonnull
public Image getImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull java.awt.Image image) throws AssetException {
return getImage(ImageSource.of(name, format, image));
}
@Nonnull
public Image getImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull Resource input) throws AssetException {
return getImage(ImageSource.of(name, format, input));
}
@Nonnull
public Image getImage(@Nonnull String path) throws AssetException {
return getImage(ImageSource.of(getResourceLoader(), path));
}
@Nonnull
public Image getImage(@Nonnull String path, @Nonnull ImageFormat format) throws AssetException {
return getImage(ImageSource.of(getResourceLoader(), path, format));
}
@Nonnull
public Image getImage(@Nonnull Url url) throws AssetException {
return getImage(ImageSource.of(url));
}
@Nonnull
public Image getImage(@Nonnull Url url, @Nonnull ImageFormat format) throws AssetException {
return getImage(ImageSource.of(url, format));
}
@Nonnull
public XView getLocalFonts() {
if (localFonts == null) {
XList list = new XArrayList<>();
for (java.awt.Font handle : GraphicsEnvironment.getLocalGraphicsEnvironment().getAllFonts()) {
list.add(loadFont(handle));
}
this.localFonts = new XBasicView<>(list);
}
return localFonts;
}
@Nonnull
public ResourceLoader getResourceLoader() {
return resourceLoader;
}
@Nonnull
public Sound getSound(@Nonnull File file) throws AssetException {
return getSound(SoundSource.of(file));
}
@Nonnull
public Sound getSound(@Nonnull File file, @Nonnull SoundFormat format) throws AssetException {
return getSound(SoundSource.of(file, format));
}
@Nonnull
public Sound getSound(@Nonnull SoundFormat format, @Nonnull byte[] input) throws AssetException {
return getSound(SoundSource.of(format, input));
}
@Nonnull
public Sound getSound(@Nonnull SoundFormat format, @Nonnull InputStream input) throws AssetException {
return getSound(SoundSource.of(format, input));
}
@Nonnull
public Sound getSound(@Nonnull SoundFormat format, @Nonnull Resource input) throws AssetException {
return getSound(SoundSource.of(format, input));
}
@Nonnull
public Sound getSound(@Nonnull SoundSource source) throws AssetException {
if (assertType(source.getName(), Sound.class)) {
Sound sound = (Sound) getCached(source.getName());
if (sound.getFormat() == source.getFormat()) {
return sound;
}
XLogger.internal("Sound cached \"" + source.getName() + "\" but different format (source: " + source.getFormat() + ", cached: " + sound.getFormat() + ')');
}
Sound sound = source.load();
insert(source.getName(), sound);
return sound;
}
@Nonnull
public Sound getSound(@Nonnull String name, @Nonnull SoundFormat format, @Nonnull byte[] input) throws AssetException {
return getSound(SoundSource.of(name, format, input));
}
@Nonnull
public Sound getSound(@Nonnull String name, @Nonnull SoundFormat format, @Nonnull InputStream input) throws AssetException {
return getSound(SoundSource.of(name, format, input));
}
@Nonnull
public Sound getSound(@Nonnull String name, @Nonnull SoundFormat format, @Nonnull Resource input) throws AssetException {
return getSound(SoundSource.of(name, format, input));
}
@Nonnull
public Sound getSound(@Nonnull String path) throws AssetException {
return getSound(SoundSource.of(getResourceLoader(), path));
}
@Nonnull
public Sound getSound(@Nonnull String path, @Nonnull SoundFormat format) throws AssetException {
return getSound(SoundSource.of(getResourceLoader(), path, format));
}
@Nonnull
public Sound getSound(@Nonnull Url url) throws AssetException {
return getSound(SoundSource.of(url));
}
@Nonnull
public Sound getSound(@Nonnull Url url, @Nonnull SoundFormat format) throws AssetException {
return getSound(SoundSource.of(url, format));
}
@Nonnull
public Font loadFont(@Nonnull File file) throws AssetException {
if (!file.exists()) {
throw new AssetException("Failed to load font: File does not exists.");
}
return loadFont(file.getPath());
}
@Nonnull
public Font loadFont(@Nonnull InputStream input) throws AssetException {
try {
return loadFont(java.awt.Font.createFont(java.awt.Font.TRUETYPE_FONT, input));
}
catch (@Nonnull FontFormatException | IOException exception) {
throw new AssetException("Failed to load font", exception);
}
}
@Nonnull
public Font loadFont(@Nonnull java.awt.Font font) {
return new TrueTypeFont(font);
}
@Nonnull
public Font loadFont(@Nonnull Resource resource) throws AssetException {
try (InputStream input = resource.newInputStream()) {
return loadFont(input);
}
catch (IOException exception) {
throw new AssetException("Failed to load font", exception);
}
}
@Nonnull
public Font loadFont(@Nonnull String path) throws AssetException {
String s = StringWorker.removeTrailingFileSeparator(path);
if (assertType(s, Font.class)) {
return (Font) getCached(s);
}
try (InputStream input = getResourceLoader().getResourceAsStream(s)) {
return loadFont(input);
}
catch (@Nonnull IOException | ResourceNotFoundException exception) {
return new TrueTypeFont(path);
}
}
@Nonnull
public Image loadIconOf(@Nonnull File file) throws AssetException {
FileSystemView view = FileSystemView.getFileSystemView();
Icon icon = view.getSystemIcon(file.handle());
java.awt.Image image = ImageWorker.asImage(icon);
return loadImage(image);
}
@Nonnull
public Image loadImage(@Nonnull ByteBuffer input, int width, int height) throws AssetException {
return loadImage(ImageSource.of(input, width, height));
}
@Nonnull
public Image loadImage(@Nonnull File file) throws AssetException {
return loadImage(ImageSource.of(file));
}
@Nonnull
public Image loadImage(@Nonnull File file, @Nonnull ImageFormat format) throws AssetException {
return loadImage(ImageSource.of(file, format));
}
@Nonnull
public Image loadImage(@Nonnull ImageFormat format, @Nonnull byte[] input) throws AssetException {
return loadImage(ImageSource.of(format, input));
}
@Nonnull
public Image loadImage(@Nonnull ImageFormat format, @Nonnull InputStream input) throws AssetException {
return loadImage(ImageSource.of(format, input));
}
@Nonnull
public Image loadImage(@Nonnull ImageFormat format, @Nonnull java.awt.Image image) throws AssetException {
return loadImage(ImageSource.of(format, image));
}
@Nonnull
public Image loadImage(@Nonnull ImageFormat format, @Nonnull Resource input) throws AssetException {
return loadImage(ImageSource.of(format, input));
}
@Nonnull
public Image loadImage(@Nonnull ImageSource source) throws AssetException {
return source.load();
}
@Nonnull
public Image loadImage(@Nonnull int[] pixels, int width, int height) throws AssetException {
return loadImage(ImageSource.of(pixels, width, height));
}
@Nonnull
public Image loadImage(@Nonnull java.awt.Image image) throws AssetException {
return loadImage(ImageSource.of(image));
}
@Nonnull
public Image loadImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull byte[] input) throws AssetException {
return loadImage(ImageSource.of(name, format, input));
}
@Nonnull
public Image loadImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull InputStream input) throws AssetException {
return loadImage(ImageSource.of(name, format, input));
}
@Nonnull
public Image loadImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull java.awt.Image image) throws AssetException {
return loadImage(ImageSource.of(name, format, image));
}
@Nonnull
public Image loadImage(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull Resource input) throws AssetException {
return loadImage(ImageSource.of(name, format, input));
}
@Nonnull
public Image loadImage(@Nonnull String path) throws AssetException {
return loadImage(ImageSource.of(getResourceLoader(), path));
}
@Nonnull
public Image loadImage(@Nonnull String path, @Nonnull ImageFormat format) throws AssetException {
return loadImage(ImageSource.of(getResourceLoader(), path, format));
}
@Nonnull
public Image loadImage(@Nonnull Url url) throws AssetException {
return loadImage(ImageSource.of(url));
}
@Nonnull
public Image loadImage(@Nonnull Url url, @Nonnull ImageFormat format) throws AssetException {
return loadImage(ImageSource.of(url, format));
}
@Nonnull
public Sound loadSound(@Nonnull File file) throws AssetException {
return loadSound(SoundSource.of(file));
}
@Nonnull
public Sound loadSound(@Nonnull File file, @Nonnull SoundFormat format) throws AssetException {
return loadSound(SoundSource.of(file, format));
}
@Nonnull
public Sound loadSound(@Nonnull SoundFormat format, @Nonnull byte[] input) throws AssetException {
return loadSound(SoundSource.of(format, input));
}
@Nonnull
public Sound loadSound(@Nonnull SoundFormat format, @Nonnull InputStream input) throws AssetException {
return loadSound(SoundSource.of(format, input));
}
@Nonnull
public Sound loadSound(@Nonnull SoundFormat format, @Nonnull Resource input) throws AssetException {
return loadSound(SoundSource.of(format, input));
}
@Nonnull
public Sound loadSound(@Nonnull SoundSource source) throws AssetException {
return source.load();
}
@Nonnull
public Sound loadSound(@Nonnull String name, @Nonnull SoundFormat format, @Nonnull byte[] input) throws AssetException {
return loadSound(SoundSource.of(name, format, input));
}
@Nonnull
public Sound loadSound(@Nonnull String name, @Nonnull SoundFormat format, @Nonnull InputStream input) throws AssetException {
return loadSound(SoundSource.of(name, format, input));
}
@Nonnull
public Sound loadSound(@Nonnull String name, @Nonnull SoundFormat format, @Nonnull Resource input) throws AssetException {
return loadSound(SoundSource.of(name, format, input));
}
@Nonnull
public Sound loadSound(@Nonnull String path) throws AssetException {
return loadSound(SoundSource.of(getResourceLoader(), path));
}
@Nonnull
public Sound loadSound(@Nonnull String path, @Nonnull SoundFormat format) throws AssetException {
return loadSound(SoundSource.of(getResourceLoader(), path, format));
}
@Nonnull
public Sound loadSound(@Nonnull Url url) throws AssetException {
return loadSound(SoundSource.of(url));
}
@Nonnull
public Sound loadSound(@Nonnull Url url, @Nonnull SoundFormat format) throws AssetException {
return loadSound(SoundSource.of(url, format));
}
public void setResourceLoader(@Nonnull ResourceLoader loader) {
this.resourceLoader = loader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy