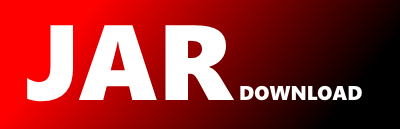
com.github.bloodshura.x.assets.AssetManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets;
import com.github.bloodshura.x.assets.exception.AssetException;
import com.github.bloodshura.x.assets.exception.CodecNotFoundException;
import com.github.bloodshura.x.assets.image.ImageFormat;
import com.github.bloodshura.x.assets.image.codec.BmpCodec;
import com.github.bloodshura.x.assets.image.codec.DefaultImageCodec;
import com.github.bloodshura.x.assets.image.codec.HdrCodec;
import com.github.bloodshura.x.assets.image.codec.ImageCodec;
import com.github.bloodshura.x.assets.image.codec.PfmCodec;
import com.github.bloodshura.x.assets.image.codec.TgaCodec;
import com.github.bloodshura.x.assets.sound.Sound;
import com.github.bloodshura.x.assets.sound.SoundFormat;
import com.github.bloodshura.x.assets.sound.codec.DefaultSoundCodec;
import com.github.bloodshura.x.assets.sound.codec.MidiCodec;
import com.github.bloodshura.x.assets.sound.codec.SoundCodec;
import com.github.bloodshura.x.assets.sound.codec.WavCodec;
import com.github.bloodshura.x.collection.map.XMap;
import com.github.bloodshura.x.collection.map.impl.XLinkedMap;
import javax.annotation.Nonnull;
import java.lang.reflect.InvocationTargetException;
public class AssetManager {
protected final XMap imageCodecs;
protected final XMap> soundCodecs;
public AssetManager() {
this.imageCodecs = new XLinkedMap<>();
this.soundCodecs = new XLinkedMap<>();
// ImageIO defaults
setCodec(ImageFormat.BMP, BmpCodec.class);
setCodec(ImageFormat.GIF, DefaultImageCodec.class);
setCodec(ImageFormat.JPG, DefaultImageCodec.class);
setCodec(ImageFormat.PNG, DefaultImageCodec.class);
setCodec(ImageFormat.WBMP, BmpCodec.class);
// Custom codecs
setCodec(ImageFormat.HDR, HdrCodec.class);
setCodec(ImageFormat.PFM, PfmCodec.class);
setCodec(ImageFormat.TGA, TgaCodec.class);
// Java Sound defaults
setCodec(SoundFormat.AIFC, DefaultSoundCodec.class);
setCodec(SoundFormat.AIFF, DefaultSoundCodec.class);
setCodec(SoundFormat.AU, DefaultSoundCodec.class);
// Custom codecs
setCodec(SoundFormat.MIDI, MidiCodec.class);
setCodec(SoundFormat.WAV, WavCodec.class);
}
@Nonnull
public SoundCodec createCodec(@Nonnull Sound sound) throws CodecNotFoundException {
try {
return getCodec(sound.getFormat()).getConstructor(Sound.class).newInstance(sound);
}
catch (IllegalAccessException | IllegalArgumentException | InstantiationException | InvocationTargetException | NoSuchMethodException | SecurityException exception) {
throw new CodecNotFoundException("Failed to create " + sound.getFormat().getName() + " codec", exception);
}
}
public void ensureCodec(@Nonnull ImageFormat format) throws CodecNotFoundException {
if (!hasCodec(format)) {
throw new CodecNotFoundException("No codec registered for " + format.getName() + " image format");
}
}
public void ensureCodec(@Nonnull SoundFormat format) throws CodecNotFoundException {
if (!hasCodec(format)) {
throw new CodecNotFoundException("No codec registered for " + format.getName() + " sound format");
}
}
@Nonnull
public ImageCodec getCodec(@Nonnull ImageFormat format) throws CodecNotFoundException {
ensureCodec(format);
return imageCodecs.get(format);
}
@Nonnull
public Class extends SoundCodec> getCodec(@Nonnull SoundFormat format) throws CodecNotFoundException {
ensureCodec(format);
return soundCodecs.get(format);
}
public boolean hasCodec(@Nonnull ImageFormat format) {
return imageCodecs.containsKey(format);
}
public boolean hasCodec(@Nonnull SoundFormat format) {
return soundCodecs.containsKey(format);
}
public void setCodec(@Nonnull ImageFormat format, @Nonnull Class extends ImageCodec> codec) throws AssetException {
if (codec != null) {
try {
setCodec(format, codec.newInstance());
}
catch (IllegalAccessException | InstantiationException exception) {
throw new AssetException("Could not call default constructor of " + codec, exception);
}
}
else {
imageCodecs.removeKey(format);
}
}
public void setCodec(@Nonnull ImageFormat format, @Nonnull ImageCodec codec) throws AssetException {
if (codec != null) {
if (!codec.hasDecoder(format) && !codec.hasEncoder(format)) {
throw new AssetException("Codec \"" + codec + "\" has neither decoder nor encoder for " + format + " format");
}
imageCodecs.set(format, codec);
}
else {
imageCodecs.removeKey(format);
}
}
public void setCodec(@Nonnull SoundFormat format, @Nonnull Class extends SoundCodec> codec) {
if (codec != null) {
soundCodecs.set(format, codec);
}
else {
soundCodecs.removeKey(format);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy