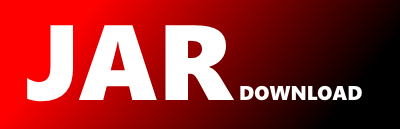
com.github.bloodshura.x.assets.font.Font Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.font;
import com.github.bloodshura.x.assets.Asset;
import com.github.bloodshura.x.assets.image.Image;
import com.github.bloodshura.x.collection.map.XMap;
import com.github.bloodshura.x.collection.map.impl.XLinkedMap;
import com.github.bloodshura.x.collection.tuple.Pair;
import com.github.bloodshura.x.lang.Nameable;
import com.github.bloodshura.x.object.storable.StorableBase;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
public abstract class Font extends StorableBase implements Asset, Nameable {
private XMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy