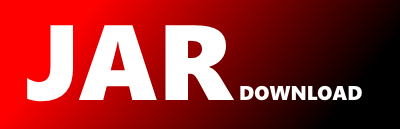
com.github.bloodshura.x.assets.font.glyph.GlyphableFont Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.font.glyph;
import com.github.bloodshura.x.assets.font.Font;
import com.github.bloodshura.x.assets.font.exception.FontException;
import javax.annotation.Nonnull;
public abstract class GlyphableFont extends Font implements Glyphable {
private final Glyph[] glyphs;
private final String name;
private final int size;
private final GlyphableFontWrapper wrapper;
public GlyphableFont(@Nonnull CharSequence name, int size) {
this.glyphs = new Glyph[maxGlyphIndex()];
this.name = name.toString();
this.size = size;
this.wrapper = new GlyphableFontWrapper(this);
}
@Nonnull
@Override
public abstract GlyphableFont clone();
@Nonnull
@Override
public Glyph getGlyph(char character) throws FontException {
if (character >= 0 && character < maxGlyphIndex()) {
Glyph glyph = getGlyphs()[character];
if (glyph != null) {
return glyph;
}
}
throw new FontException("Character \"" + character + "\" not supported");
}
@Nonnull
public Glyph[] getGlyphs() {
return glyphs;
}
@Nonnull
@Override
public String getName() {
return name;
}
@Override
public int getSize() {
return size;
}
@Override
public int getWidth(@Nonnull CharSequence s) {
int sum = 0;
for (int i = 0; i < s.length(); i++) {
char ch = s.charAt(i);
if (isSupported(ch)) {
Glyph glyph = getGlyph(ch);
sum += glyph.getWidth();
}
}
return sum;
}
@Override
public boolean isSupported(char character) {
try {
getGlyph(character);
return true;
}
catch (FontException exception) {
return false;
}
}
public int maxGlyphIndex() {
return 256;
}
// TODO Rework dis shet with the wrapper; 28-10-2015
@Nonnull
@Override
public String[] wrapString(@Nonnull String s, int width) {
return wrapper.wrapStringToWidth(s, width).split(String.valueOf((char) 32767));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy