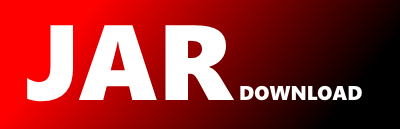
com.github.bloodshura.x.assets.image.ImageFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.image;
import com.github.bloodshura.x.assets.AssetFormat;
import com.github.bloodshura.x.assets.AssetManager;
import com.github.bloodshura.x.assets.XAssets;
import com.github.bloodshura.x.collection.list.XList;
import com.github.bloodshura.x.collection.list.impl.XLinkedList;
import com.github.bloodshura.x.collection.view.XArrayView;
import com.github.bloodshura.x.collection.view.XBasicView;
import com.github.bloodshura.x.collection.view.XView;
import com.github.bloodshura.x.enumeration.Enumerations;
import javax.annotation.Nonnull;
// NÃO ALTERAR A ORDEM DOS MIMETYPES. OS PRIMEIROS SÃO USADOS PELO ShuraX Assets Extensions.
public class ImageFormat extends AssetFormat {
public static final ImageFormat APF = new ImageFormat("APF", new String[] { "apf" }, "image/apf");
public static final ImageFormat BMP = new ImageFormat("BMP", new String[] { "bmp", "dib" }, "image/bmp", "image/x-bmp", "image/x-bitmap", "image/x-xbitmap", "image/x-win-bitmap", "image/x-windows-bmp", "image/ms-bmp", "image/x-ms-bmp");
public static final ImageFormat CUR = new ImageFormat("CUR", new String[] { "cur" }, "image/cur", "image/x-win-bitmap");
public static final ImageFormat GIF = new ImageFormat("GIF", new String[] { "gif" }, "image/gif", "image/x-xbitmap");
public static final ImageFormat HDR = new ImageFormat("HDR", new String[] { "hdr" }, "image/hdr");
public static final ImageFormat ICO = new ImageFormat("ICO", new String[] { "ico" }, "image/ico", "image/x-icon", "image/x-win-bitmap");
public static final ImageFormat JPG = new ImageFormat("JPG", new String[] { "jpg", "jpeg" }, "image/jpg", "image/jpeg", "image/pjpeg", "image/pipeg", "image/vnd.swiftview-jpeg", "image/x-xbitmap");
public static final ImageFormat PCT = new ImageFormat("PCT", new String[] { "pct", "pict" }, "image/pict");
public static final ImageFormat PCX = new ImageFormat("PCX", new String[] { "pcx" }, "image/pcx", "image/x-pcx", "image/x-pc-paintbrush");
public static final ImageFormat PFM = new ImageFormat("PFM", new String[] { "pfm" }, "image/pfm");
public static final ImageFormat PNG = new ImageFormat("PNG", new String[] { "png" }, "image/png");
public static final ImageFormat PSD = new ImageFormat("PSD", new String[] { "psd" }, "image/psd", "image/photoshop", "image/x-photoshop");
public static final ImageFormat RAS = new ImageFormat("RAS", new String[] { "ras" }, "image/cmu-raster", "image/ras");
public static final ImageFormat TGA = new ImageFormat("TGA", new String[] { "tga" }, "image/targa", "image/tga", "image/x-targa", "image/x-tga");
public static final ImageFormat TIFF = new ImageFormat("TIFF", new String[] { "tiff", "tif" }, "image/tiff");
public static final ImageFormat WBMP = new ImageFormat("WBMP", new String[] { "wbmp" }, "image/vnd.wap.wbmp", "image/wbmp");
public static final ImageFormat XBM = new ImageFormat("XBM", new String[] { "xbm" }, "image/xbm", "image/x-xbitmap");
public static final ImageFormat XPM = new ImageFormat("XPM", new String[] { "xpm" }, "image/xpm", "image/x-xbitmap", "image/x-xpixmap", "image/x-xpm");
private final XView mimeTypes;
public ImageFormat(@Nonnull String name, @Nonnull String[] formats, @Nonnull String... mimeTypes) {
super(name, formats);
this.mimeTypes = new XArrayView<>(mimeTypes);
}
@Nonnull
public XView getMimeTypes() {
return mimeTypes;
}
@Nonnull
public static XView byMimeType(@Nonnull CharSequence s) {
String str = s.toString();
XList list = new XLinkedList<>();
values().forEach(format -> format.getMimeTypes().forEach(mimeType -> {
if (mimeType.equalsIgnoreCase(str)) {
list.add(format);
}
}));
return new XBasicView<>(list);
}
@Nonnull
public static XView readableFormats() {
return readableFormats(XAssets.getManager());
}
@Nonnull
public static XView readableFormats(@Nonnull AssetManager manager) {
return values().select(format -> manager.hasCodec(format) && manager.getCodec(format).hasDecoder(format));
}
@Nonnull
public static XView values() {
return Enumerations.values(ImageFormat.class);
}
@Nonnull
public static XView writableFormats() {
return writableFormats(XAssets.getManager());
}
@Nonnull
public static XView writableFormats(@Nonnull AssetManager manager) {
return values().select(format -> manager.hasCodec(format) && manager.getCodec(format).hasEncoder(format));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy