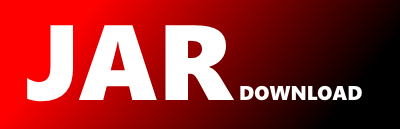
com.github.bloodshura.x.assets.image.ImageSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.image;
import com.github.bloodshura.x.assets.AssetSource;
import com.github.bloodshura.x.assets.exception.AssetException;
import com.github.bloodshura.x.assets.image.source.AWTImageSource;
import com.github.bloodshura.x.assets.image.source.InputImageSource;
import com.github.bloodshura.x.assets.util.ImageWorker;
import com.github.bloodshura.x.enumeration.Enumerations;
import com.github.bloodshura.x.io.Url;
import com.github.bloodshura.x.io.file.File;
import com.github.bloodshura.x.loader.ResourceLoader;
import com.github.bloodshura.x.resource.ByteArrayDirectResource;
import com.github.bloodshura.x.resource.PathResource;
import com.github.bloodshura.x.resource.Resource;
import com.github.bloodshura.x.worker.StringWorker;
import javax.annotation.Nonnull;
import java.io.InputStream;
import java.nio.ByteBuffer;
public abstract class ImageSource extends AssetSource {
private static int UNNAMED_COUNT = 0;
public ImageSource(@Nonnull String name, @Nonnull ImageFormat format) {
super(name, format);
}
@Nonnull
public static ImageSource of(@Nonnull ByteBuffer buffer, int width, int height) {
return of(ImageWorker.asImage(buffer, width, height));
}
@Nonnull
public static ImageSource of(@Nonnull File file) throws AssetException {
ImageFormat format = Enumerations.search(ImageFormat.class, file.getFormat());
if (format != null) {
return of(file, format);
}
throw new AssetException("Could not define image format for \"" + file.getPath() + "\"");
}
@Nonnull
public static ImageSource of(@Nonnull File file, @Nonnull ImageFormat format) {
return of(file.getPath(), format, file);
}
@Nonnull
public static ImageSource of(@Nonnull ImageFormat format, @Nonnull byte[] input) {
return of(unnamed(), format, input);
}
@Nonnull
public static ImageSource of(@Nonnull ImageFormat format, @Nonnull InputStream input) {
return of(unnamed(), format, input);
}
@Nonnull
public static ImageSource of(@Nonnull ImageFormat format, @Nonnull Resource input) {
return of(unnamed(), format, input);
}
@Nonnull
public static ImageSource of(@Nonnull ImageFormat format, @Nonnull java.awt.Image image) {
return of(unnamed(), format, image);
}
@Nonnull
public static ImageSource of(@Nonnull int[] pixels, int width, int height) {
return of(ImageWorker.generateImage(width, height, pixels));
}
@Nonnull
public static ImageSource of(@Nonnull java.awt.Image image) {
return of(ImageFormat.PNG, image);
}
@Nonnull
public static ImageSource of(@Nonnull ResourceLoader loader, @Nonnull String path) throws AssetException {
String s = StringWorker.removeTrailingFileSeparator(path);
int dot = s.lastIndexOf('.');
if (dot == -1 || dot >= s.length() - 1) {
throw new AssetException("Could not find format for \"" + s + "\"");
}
String formatStr = s.substring(dot + 1);
int sep = formatStr.indexOf('?');
if (sep != -1) {
formatStr = formatStr.substring(0, sep);
}
ImageFormat format = Enumerations.search(ImageFormat.class, formatStr);
if (format != null) {
return of(loader, path, format);
}
throw new AssetException("Invalid image format \"" + formatStr + "\"");
}
@Nonnull
public static ImageSource of(@Nonnull ResourceLoader loader, @Nonnull String path, @Nonnull ImageFormat format) throws AssetException {
return of(path, format, new PathResource(loader, path));
}
@Nonnull
public static ImageSource of(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull byte[] input) {
return of(name, format, new ByteArrayDirectResource(input));
}
@Nonnull
public static ImageSource of(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull InputStream input) {
return of(name, format, () -> input);
}
@Nonnull
public static ImageSource of(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull Resource input) {
return new InputImageSource(name, format, input);
}
@Nonnull
public static ImageSource of(@Nonnull String name, @Nonnull ImageFormat format, @Nonnull java.awt.Image image) {
return new AWTImageSource(name, format, ImageWorker.asBufferedImage(image));
}
@Nonnull
public static ImageSource of(@Nonnull Url url) throws AssetException {
if (url.hasFormat()) {
ImageFormat format = Enumerations.search(ImageFormat.class, url.getFormat());
if (format != null) {
return of(url, format);
}
}
throw new AssetException("Could not define image format for \"" + url.getPath() + "\"");
}
@Nonnull
public static ImageSource of(@Nonnull Url url, @Nonnull ImageFormat format) {
return of(url.getPath(), format, url);
}
@Nonnull
protected static String unnamed() {
return "XImage-" + UNNAMED_COUNT++;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy