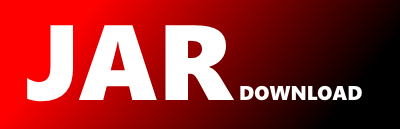
com.github.bloodshura.x.assets.image.codec.PfmCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.image.codec;
import com.github.bloodshura.x.assets.image.ImageFormat;
import com.github.bloodshura.x.assets.util.ImageWorker;
import com.github.bloodshura.x.memory.Bufferer;
import com.github.bloodshura.x.worker.ParseWorker;
import com.github.bloodshura.x.worker.StringWorker;
import javax.annotation.Nonnull;
import java.awt.image.BufferedImage;
import java.io.DataInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
// TODO Who's the author?!
public class PfmCodec implements ImageCodec {
@Override
public boolean hasDecoder(@Nonnull ImageFormat format) {
return format == ImageFormat.PFM;
}
@Override
public boolean hasEncoder(@Nonnull ImageFormat format) {
return false;
}
@Nonnull
@Override
public BufferedImage read(@Nonnull InputStream input) throws IOException {
DataInputStream dataInput = new DataInputStream(input);
String format = dataInput.readUTF();
int bytesPerPixel;
if (format.equals("PF")) {
bytesPerPixel = 12;
}
else if (format.equals("Pf")) {
bytesPerPixel = 4;
}
else {
throw new IOException("File is not in PFM format");
}
String sizeStr = dataInput.readUTF();
if (!StringWorker.contains(sizeStr, ' ')) {
throw new IOException("Corrupt or invalid PFM file: invalid dimensions header");
}
String[] split = sizeStr.split(" ");
int width = ParseWorker.toInt(split[0]);
int height = ParseWorker.toInt(split[1]);
if (width <= 0 || height <= 0) {
throw new IOException("Corrupt or invalid PFM file: invalid dimensions header");
}
String scaleStr = dataInput.readUTF();
float scale = ParseWorker.toFloat(scaleStr);
ByteOrder order = scale < 0 ? ByteOrder.LITTLE_ENDIAN : ByteOrder.BIG_ENDIAN;
boolean needsEndianFlip = order != ByteOrder.nativeOrder();
int scanLineBytes = bytesPerPixel * width;
ByteBuffer buffer = Bufferer.newByteBuffer(width * height * bytesPerPixel);
byte[] scanLine = new byte[width * bytesPerPixel];
for (int y = height - 1; y >= 0; y--) {
int read;
int off = 0;
buffer.position(scanLineBytes * y);
do {
read = input.read(scanLine, off, scanLine.length - off);
off += read;
}
while (read > 0);
if (needsEndianFlip) {
for (int i = 0; i < scanLine.length; i += 4) {
byte data = scanLine[i + 3];
scanLine[i + 3] = scanLine[i];
scanLine[i] = data;
data = scanLine[i + 2];
scanLine[i + 2] = scanLine[i + 1];
scanLine[i + 1] = data;
}
}
buffer.put(scanLine);
}
buffer.rewind();
return ImageWorker.asImage(buffer, width, height);
}
@Deprecated
@Override
public void write(@Nonnull BufferedImage image, @Nonnull ImageFormat format, @Nonnull OutputStream output) {
throw new UnsupportedOperationException("PFM encoding not supported");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy