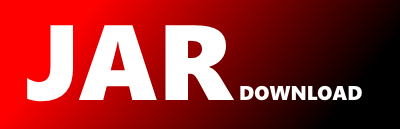
com.github.bloodshura.x.assets.sound.SoundInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.sound;
import com.github.bloodshura.x.assets.sound.exception.SoundException;
import com.github.bloodshura.x.collection.map.impl.XLinkedMap;
import com.github.bloodshura.x.collection.tuple.Pair;
import com.github.bloodshura.x.collection.view.XBasicView;
import com.github.bloodshura.x.collection.view.XView;
import com.github.bloodshura.x.io.file.File;
import com.github.bloodshura.x.memory.Bytes;
import com.github.bloodshura.x.object.Base;
import com.github.bloodshura.x.worker.ParseWorker;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.sound.sampled.AudioFileFormat;
import javax.sound.sampled.AudioFormat;
import javax.sound.sampled.AudioSystem;
import javax.sound.sampled.UnsupportedAudioFileException;
import java.io.IOException;
import java.time.Duration;
import java.time.LocalDate;
import java.time.Month;
import java.util.concurrent.TimeUnit;
public class SoundInfo extends Base {
private final File file;
private final AudioFileFormat infoFormat;
private final XView> properties;
public SoundInfo(@Nonnull File file) throws SoundException {
this.file = file;
try {
this.infoFormat = AudioSystem.getAudioFileFormat(getFile().handle());
this.properties = new XBasicView<>(new XLinkedMap<>(infoFormat.properties()));
}
catch (IOException exception) {
throw new SoundException(exception);
}
catch (UnsupportedAudioFileException exception) {
throw new SoundException("Unsupported audio file: " + file.getFullName() + " [" + file.getFormat() + ']');
}
}
@Nullable
public String getAlbum() {
return getProperty("album");
}
@Nullable
public String getArtist() {
return getProperty("author");
}
@Nullable
public String getComment() {
return getProperty("comment");
}
@Nullable
public String getCopyright() {
return getProperty("copyright");
}
@Nullable
public LocalDate getDate() {
String year = getProperty("date");
if (year != null && ParseWorker.isInt(year)) {
return LocalDate.of(ParseWorker.toInt(year), Month.JANUARY, 1);
}
return null;
}
@Nullable
public Duration getDuration() {
if (infoFormat.properties().containsKey("duration")) {
long microDuration = (long) infoFormat.properties().get("duration");
return Duration.ofMillis(TimeUnit.MICROSECONDS.toMillis(microDuration));
}
return null;
}
@Nonnull
public File getFile() {
return file;
}
@Nonnull
public AudioFormat getFormat() {
return infoFormat.getFormat();
}
@Nonnull
public Bytes getFrameLength() {
return new Bytes(infoFormat.getFrameLength());
}
@Nullable
public String getProperty(String key) {
return hasProperty(key) ? infoFormat.properties().get(key).toString() : null;
}
@Nonnull
public Bytes getSize() {
return new Bytes(infoFormat.getByteLength());
}
@Nullable
public String getTitle() {
return getProperty("title");
}
public boolean hasAlbum() {
return getAlbum() != null;
}
public boolean hasArtist() {
return getArtist() != null;
}
public boolean hasComment() {
return getComment() != null;
}
public boolean hasCopyright() {
return getCopyright() != null;
}
public boolean hasDate() {
return getDate() != null;
}
public boolean hasDuration() {
return getDuration() != null;
}
public boolean hasProperty(@Nonnull String key) {
return infoFormat.properties().containsKey(key);
}
public boolean hasTitle() {
return getTitle() != null;
}
@Nonnull
public XView> properties() {
return properties;
}
@Nonnull
@Override
protected Object[] stringValues() {
return new Object[] { getSize(), getFrameLength(), properties() };
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy