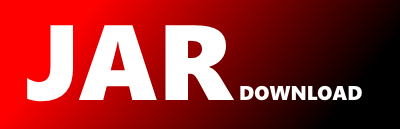
com.github.bloodshura.x.assets.sound.codec.MidiCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.sound.codec;
import com.github.bloodshura.x.assets.sound.Sound;
import com.github.bloodshura.x.assets.sound.Sound.PlayMode;
import com.github.bloodshura.x.assets.sound.exception.SoundException;
import javax.annotation.Nonnull;
import javax.sound.midi.InvalidMidiDataException;
import javax.sound.midi.MidiSystem;
import javax.sound.midi.MidiUnavailableException;
import javax.sound.midi.Sequence;
import javax.sound.midi.Sequencer;
import java.io.IOException;
import java.time.Duration;
import java.util.concurrent.TimeUnit;
public class MidiCodec implements SoundCodec {
private Sequence sequence;
private Sequencer sequencer;
private final Sound sound;
public MidiCodec(@Nonnull Sound sound) {
this.sound = sound;
}
@Nonnull
@Override
public Duration getDuration() {
return Duration.ofNanos(TimeUnit.MICROSECONDS.toNanos(sequence.getMicrosecondLength()));
}
@Deprecated
@Override
public double getGain() {
return 1.0D;
}
@Deprecated
@Override
public double getPitch() {
return 1.0D;
}
@Nonnull
@Override
public Duration getPosition() {
if (isPlaying()) {
return Duration.ofNanos(TimeUnit.MICROSECONDS.toNanos(sequencer.getMicrosecondPosition()));
}
return null;
}
@Override
public boolean isPlaying() {
return sequencer.getSequence() == sequence && sequencer.isRunning();
}
@Override
public boolean isSetUp() {
return sequence != null && sequencer != null;
}
@Override
public void play(@Nonnull PlayMode playMode) throws SoundException {
if (!isPlaying()) {
try {
sequencer.setSequence(sequence);
sequencer.setLoopCount(playMode == PlayMode.LOOP ? Integer.MAX_VALUE : 0);
sequencer.start();
}
catch (InvalidMidiDataException exception) {
throw new SoundException(exception.getMessage());
}
}
}
@Deprecated
@Override
public void setGain(double gain) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@Deprecated
@Override
public void setPitch(double pitch) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@Override
public void setPosition(@Nonnull Duration duration) {
sequencer.setMicrosecondPosition(TimeUnit.NANOSECONDS.toMicros(duration.toNanos()));
}
@Override
public void setup() throws IOException {
try {
this.sequence = MidiSystem.getSequence(sound.newInputStream());
this.sequencer = MidiSystem.getSequencer();
sequencer.open();
}
catch (InvalidMidiDataException | MidiUnavailableException exception) {
// These exceptions are only constructable with message, without cause, so no need to pass it.
throw new IOException(exception.getMessage());
}
}
@Override
public void stop() {
if (isPlaying()) {
sequencer.stop();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy