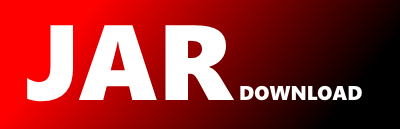
com.github.bloodshura.x.assets.util.ImageWorker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-assets Show documentation
Show all versions of shurax-assets Show documentation
An API for reading, writing and manipulating fonts, images and sounds.
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.assets.util;
import com.github.bloodshura.x.assets.XAssets;
import com.github.bloodshura.x.assets.image.Image;
import com.github.bloodshura.x.color.Color;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import java.awt.Component;
import java.awt.Container;
import java.awt.Cursor;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.nio.ByteBuffer;
// TODO Make some cleanup on this shet // 13-08-2015
public class ImageWorker {
//public static static final int[] BIT_MASKS = new int[] { 0xFF0000, 0xFF00, 0xFF, 0xFF000000 };
public static final int DEFAULT_TYPE = BufferedImage.TYPE_INT_ARGB;
public static void applyGlobalCursor(@Nonnull Container container, @Nullable Cursor cursor) {
container.setCursor(cursor);
for (Component component : container.getComponents()) {
if (component instanceof Container) {
applyGlobalCursor((Container) component, cursor);
}
else {
component.setCursor(cursor);
}
}
}
public static void applyGlobalCursor(@Nonnull Container container, @Nonnull Image image) {
applyGlobalCursor(container, image.toCursor());
}
@Nonnull
public static BufferedImage asBufferedImage(@Nonnull java.awt.Image image) {
return asBufferedImage(image, DEFAULT_TYPE);
}
@Nonnull
public static BufferedImage asBufferedImage(@Nonnull java.awt.Image image, int type) {
if (image instanceof BufferedImage) {
return (BufferedImage) image;
}
image = new ImageIcon(image).getImage();
if (type == BufferedImage.TYPE_CUSTOM) {
type = DEFAULT_TYPE;
}
BufferedImage bufferedImage = new BufferedImage(image.getWidth(null), image.getHeight(null), type);
Graphics graphics = bufferedImage.createGraphics();
graphics.drawImage(image, 0, 0, null);
graphics.dispose();
return bufferedImage;
}
@Nonnull
public static float[] asHSB(@Nonnull Color color) {
return asHSB(color.getRed(), color.getGreen(), color.getBlue());
}
@Nonnull
public static float[] asHSB(int red, int green, int blue) {
return java.awt.Color.RGBtoHSB(red, green, blue, null);
}
@Nonnull
public static float[] asHSB(@Nonnull int[] rgb) {
return asHSB(rgb[0], rgb[1], rgb[2]);
}
public static int asHash(int red, int green, int blue, int alpha) {
return (alpha & 0xFF) << 24 | (red & 0xFF) << 16 | (green & 0xFF) << 8 | blue & 0xFF;
}
@Nonnull
public static BufferedImage asImage(@Nonnull ByteBuffer pixels, int width, int height) {
int bpp = pixels.capacity() / (width * height);
int[] packedPixels = new int[width * height * bpp];
int bufferInd = 0;
for (int y = height - 1; y >= 0; y--) {
for (int x = 0; x < width; x++) {
int index = (y * width + x) * bpp;
packedPixels[index++] = pixels.get(bufferInd++);
packedPixels[index++] = pixels.get(bufferInd++);
if (bpp >= 4) {
packedPixels[index++] = pixels.get(bufferInd++);
packedPixels[index] = pixels.get(bufferInd++);
}
else {
packedPixels[index] = pixels.get(bufferInd++);
}
}
}
BufferedImage image = new BufferedImage(width, height, DEFAULT_TYPE);
image.getRaster().setPixels(0, 0, width, height, packedPixels);
return image;
}
@Nonnull
public static BufferedImage asImage(@Nonnull Icon icon) {
if (icon instanceof ImageIcon) {
ImageIcon imageIcon = (ImageIcon) icon;
return asBufferedImage(imageIcon.getImage());
}
BufferedImage image = new BufferedImage(icon.getIconWidth(), icon.getIconHeight(), DEFAULT_TYPE);
Graphics2D graphics = image.createGraphics();
icon.paintIcon(null, graphics, 0, 0);
graphics.dispose();
return image;
}
@Nonnull
public static BufferedImage asImageFromHashes(@Nonnull int[] pixels, int width, int height) {
BufferedImage image = new BufferedImage(width, height, DEFAULT_TYPE);
int[] packedPixels = new int[pixels.length * 4];
for (int i = 0; i < packedPixels.length; ) {
int[] rgb = asRGBA(pixels[i / 4]);
packedPixels[i++] = rgb[0];
packedPixels[i++] = rgb[1];
packedPixels[i++] = rgb[2];
packedPixels[i++] = rgb[3];
}
image.getRaster().setPixels(0, 0, width, height, packedPixels);
return image;
}
@Nonnull
public static int[] asRGBA(int hash) {
int[] rgba = new int[4];
asRGBA(hash, rgba);
return rgba;
}
public static void asRGBA(int hash, @Nonnull int[] rgba) {
rgba[0] = hash >> 16 & 0xFF;
rgba[1] = hash >> 8 & 0xFF;
rgba[2] = hash & 0xFF;
rgba[3] = hash >> 24 & 0xFF;
if (rgba[3] == 0x0) {
//rgba[3] = 0xFF; TODO ... 12-01-2018
}
}
@Nonnull
public static BufferedImage cloneImage(@Nonnull BufferedImage image) {
BufferedImage result = new BufferedImage(image.getWidth(), image.getHeight(), image.getType() != BufferedImage.TYPE_CUSTOM ? image.getType() : DEFAULT_TYPE);
Graphics2D graphics = result.createGraphics();
graphics.drawImage(image, 0, 0, null);
graphics.dispose();
return result;
}
@Nonnull
public static BufferedImage generateImage(int width, int height, @Nonnull int[] pixels) {
/*
SinglePixelPackedSampleModel parser = new SinglePixelPackedSampleModel(DataBuffer.TYPE_INT, width, height, BIT_MASKS);
DataBufferInt buffer = new DataBufferInt(pixels, pixels.length);
WritableRaster raster = Raster.createWritableRaster(parser, buffer, new Point());
return new BufferedImage(ColorModel.getRGBdefault(), raster, false, null);
*/
BufferedImage image = new BufferedImage(width, height, DEFAULT_TYPE);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, pixels[(y * width) + x]);
}
}
return image;
}
public static int indexValue(@Nonnull Number value, int max) {
int val;
if (value instanceof Double || value instanceof Float) {
val = (int) (value.doubleValue() * max);
}
else {
val = value.intValue();
}
return Math.max(0, Math.min(val, max));
}
@Nonnull
public static Image join(@Nonnull Image... images) {
BufferedImage result = new BufferedImage(images[0].getWidth(), images[0].getHeight(), images[0].getImage().getType());
Graphics2D graphics = result.createGraphics();
for (Image image : images) {
graphics.drawImage(image.getImage(), 0, 0, null);
}
graphics.dispose();
return XAssets.getLoader().getImage(result);
}
public static int truncate(int value) {
return Math.max(0, Math.min(255, value));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy