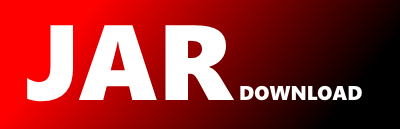
com.github.bloodshura.x.utilities.XRobot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-utilities Show documentation
Show all versions of shurax-utilities Show documentation
A collection of general utilities.
The newest version!
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.utilities;
import com.github.bloodshura.x.assets.XAssets;
import com.github.bloodshura.x.assets.image.Image;
import com.github.bloodshura.x.color.Color;
import com.github.bloodshura.x.geometry.bounds.IBounds;
import com.github.bloodshura.x.geometry.position.IPosition;
import com.github.bloodshura.x.geometry.position.Position;
import com.github.bloodshura.x.input.Key;
import com.github.bloodshura.x.input.MouseButton;
import com.github.bloodshura.x.sys.XSystem;
import javax.annotation.Nonnull;
import java.awt.AWTException;
import java.awt.Rectangle;
import java.awt.Robot;
public final class XRobot {
private static Robot robot;
@Nonnull
public static Image captureScreen() throws UnsupportedOperationException {
return captureScreen(XSystem.getDesktop().getResolution());
}
@Nonnull
public static Image captureScreen(@Nonnull IBounds bounds) throws UnsupportedOperationException {
return captureScreen(new Position(), bounds);
}
@Nonnull
public static Image captureScreen(@Nonnull IPosition position, @Nonnull IBounds bounds) throws UnsupportedOperationException {
Rectangle rectangle = new Rectangle((int) position.getX(), (int) position.getY(), (int) bounds.getWidth(), (int) bounds.getHeight());
return captureScreen(rectangle);
}
@Nonnull
public static Image captureScreen(@Nonnull Rectangle rectangle) throws UnsupportedOperationException {
return XAssets.getLoader().getImage(handle().createScreenCapture(rectangle));
}
@Nonnull
public static Robot handle() throws UnsupportedOperationException {
if (robot == null) {
try {
XRobot.robot = new Robot();
}
catch (AWTException exception) {
throw new UnsupportedOperationException("Could not create a new robot instance", exception);
}
}
return robot;
}
public static void holdButton(@Nonnull MouseButton button) throws UnsupportedOperationException {
if (!button.isAccepted()) {
throw new UnsupportedOperationException("Current toolkit does not accepts non-standard mouse button \"" + button + '"');
}
handle().mousePress(button.getMask());
}
public static void holdKey(@Nonnull Key key) throws UnsupportedOperationException {
handle().keyPress(key.getCode());
}
public static void moveMouse(int x, int y) throws UnsupportedOperationException {
handle().mouseMove(x, y);
}
public static void moveMouse(@Nonnull IPosition position) throws UnsupportedOperationException {
moveMouse((int) position.getX(), (int) position.getY());
}
@Nonnull
public static Color pixelAt(int x, int y) throws UnsupportedOperationException {
return new Color(handle().getPixelColor(x, y));
}
@Nonnull
public static Color pixelAt(@Nonnull IPosition position) throws UnsupportedOperationException {
return pixelAt((int) position.getX(), (int) position.getY());
}
public static void pressButton(@Nonnull MouseButton button) throws UnsupportedOperationException {
holdButton(button);
releaseButton(button);
}
public static void pressKey(@Nonnull Key key) throws UnsupportedOperationException {
holdKey(key);
releaseKey(key);
}
public static void releaseButton(@Nonnull MouseButton button) throws UnsupportedOperationException {
if (!button.isAccepted()) {
throw new UnsupportedOperationException("Current toolkit does not accepts non-standard mouse button \"" + button + '"');
}
handle().mouseRelease(button.getMask());
}
public static void releaseKey(@Nonnull Key key) throws UnsupportedOperationException {
handle().keyRelease(key.getCode());
}
public static void setWaitsForIdle(boolean waitsForIdle) throws UnsupportedOperationException {
handle().setAutoWaitForIdle(waitsForIdle);
}
public static boolean waitsForIdle() throws UnsupportedOperationException {
return handle().isAutoWaitForIdle();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy