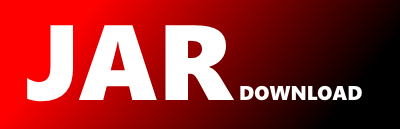
com.github.bloodshura.x.utilities.eval.Expression Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shurax-utilities Show documentation
Show all versions of shurax-utilities Show documentation
A collection of general utilities.
The newest version!
/*
* Copyright (c) 2013-2018, João Vitor Verona Biazibetti - All Rights Reserved
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* https://www.github.com/BloodShura
*/
package com.github.bloodshura.x.utilities.eval;
import com.github.bloodshura.x.object.Base;
import com.github.bloodshura.x.util.XApi;
import com.github.bloodshura.x.utilities.eval.compiler.Compiler;
import com.github.bloodshura.x.utilities.eval.compiler.Tokenizer;
import com.github.bloodshura.x.utilities.eval.exception.EvaluationException;
import com.github.bloodshura.x.utilities.eval.operation.Operation;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.math.BigDecimal;
public class Expression extends Base {
private Operation compiled;
private String input;
private VariableMap variableMap;
public Expression() {
this(null);
}
public Expression(@Nullable CharSequence input) {
this(input, new VariableMap());
}
public Expression(@Nullable CharSequence input, @Nonnull VariableMap variableMap) {
setInput(input);
setVariableMap(variableMap);
}
public void addVariables(@Nonnull Object... variables) throws EvaluationException {
addVariables(variables, 0, variables.length);
}
public void addVariables(@Nonnull Object[] variables, int offset, int length) throws EvaluationException {
XApi.require(length % 2 == 0, "Object array length must be multiple of 2 (key, value, ...)");
for (int i = offset; i < offset + length; i += 2) {
Object key = variables[i];
Object value = variables[i + 1];
XApi.require(key instanceof String, "Expected argument " + i + " to be a String; received " + key.getClass().getName());
String string = (String) key;
if (value instanceof BigDecimal) {
getVariableMap().set(string, (BigDecimal) value);
}
else if (value instanceof Number) {
Number number = (Number) value;
if (number instanceof Double || number instanceof Float) {
getVariableMap().set(string, BigDecimal.valueOf(number.doubleValue()));
}
else {
getVariableMap().set(string, BigDecimal.valueOf(number.longValue()));
}
}
else if (value instanceof String) {
getVariableMap().set(string, (String) value);
}
else {
XApi.raise("Expected argument " + i + " to be a BigDecimal, Number or String; received " + value.getClass().getName());
}
}
}
public void compile() throws EvaluationException {
if (isCompiled()) {
return;
}
XApi.requireState(hasInput(), "No input set");
Tokenizer tokenizer = new Tokenizer(getInput());
this.compiled = Compiler.compile(tokenizer);
}
@Nonnull
public BigDecimal evaluate() throws EvaluationException {
compile();
return compiled.evaluate(getVariableMap());
}
@Nullable
public String getInput() {
return input;
}
@Nonnull
public VariableMap getVariableMap() {
return variableMap;
}
public boolean hasInput() {
return getInput() != null;
}
public boolean isCompiled() {
return compiled != null;
}
public void setInput(@Nullable CharSequence input) {
this.compiled = null;
this.input = input != null ? input.toString() : null;
}
public void setVariableMap(@Nonnull VariableMap variableMap) {
this.variableMap = variableMap;
}
@Nonnull
@Override
public String toString() {
return hasInput() ? getInput() : "";
}
@Nonnull
@Override
protected Object[] stringValues() {
return new Object[] { getInput() };
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy