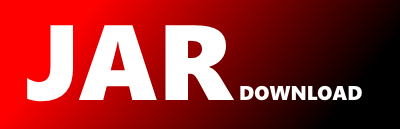
com.github.bloodshura.sparkium.mail.guerrilla.GuerrillaService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sparkium-mail Show documentation
Show all versions of sparkium-mail Show documentation
An API which aims to facilitate the usage of javax.mail, also including other mailing services.
The newest version!
package com.github.bloodshura.sparkium.mail.guerrilla;
import com.github.bloodshura.ignitium.activity.logging.XLogger;
import com.github.bloodshura.ignitium.cfg.json.JsonArray;
import com.github.bloodshura.ignitium.cfg.json.JsonObject;
import com.github.bloodshura.ignitium.cfg.json.JsonParseException;
import com.github.bloodshura.ignitium.cfg.json.JsonType;
import com.github.bloodshura.ignitium.collection.list.XList;
import com.github.bloodshura.ignitium.collection.list.impl.XArrayList;
import com.github.bloodshura.ignitium.collection.view.XBasicView;
import com.github.bloodshura.ignitium.collection.view.XView;
import com.github.bloodshura.ignitium.http.HttpMethod;
import com.github.bloodshura.ignitium.http.HttpMethod.Type;
import com.github.bloodshura.ignitium.http.QueryProperties;
import com.github.bloodshura.ignitium.http.response.PlainQueryResponse;
import com.github.bloodshura.ignitium.io.Url;
import com.github.bloodshura.ignitium.net.LocalAddress;
import com.github.bloodshura.ignitium.resource.StringResource;
import com.github.bloodshura.ignitium.worker.ParseWorker;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.LocalTime;
public class GuerrillaService {
private final Cookies cookies;
private GuerrillaEmail email;
private int lastEmailId;
/**
* Constructs a new GuerrillaMail service instance.
*/
public GuerrillaService() {
this.cookies = new Cookies();
}
/**
* Creates a new get method to the specified function name.
*
* @param functionName The function name, ex. "get_email_address"
* @return {@link HttpMethod}
*/
@Nonnull
public HttpMethod createGet(@Nonnull String functionName) {
Url url = new Url("http://api.guerrillamail.com/ajax.php");
HttpMethod method = new HttpMethod(url, Type.GET);
method.getHeaders().add("Cookie", getCookies().build());
populateContent(method.getParameters(), functionName);
return method;
}
public void deleteEmail() throws IOException {
if (hasEmail()) {
HttpMethod method = createGet("forget_me");
method.getParameters().add("email_addr", getEmail().getAddress());
method.execute();
}
this.lastEmailId = 0;
getCookies().setSubscr(null);
}
/**
* Requests the server the content of the specified message.
*
* @param message The {@link GuerrillaMessage}
* @return String with the content, or NULL if the response code was not OK (200) or the JSON was invalid
* @throws IOException
*/
@Nullable
public String getContent(@Nonnull GuerrillaMessage message) throws IOException {
HttpMethod method = createGet("fetch_email");
method.getParameters().add("email_id", message.getId());
JsonObject object = retrieve(method);
if (object == null) {
return null;
}
return object.getString("mail_body");
}
/**
* Gets the Cookies unique and final instance.
*
* @return {@link Cookies}
*/
@Nonnull
public Cookies getCookies() {
return cookies;
}
/**
* Gets the local field email.
*
* @return TempEmail
*/
@Nullable
public GuerrillaEmail getEmail() {
return email;
}
/**
* Checks if there is an already set email on local field.
*
* @return boolean
*/
public boolean hasEmail() {
return getEmail() != null;
}
/**
* Requests a new email for the server, clearing all {@link #cookies} and session data.
* Sets the new email received into the local field {@link #email}.
*
* @throws IOException
*/
public void newEmail() throws IOException {
getCookies().setPhpSessId(null);
getCookies().setSubscr(null);
this.email = retrieveEmail();
this.lastEmailId = 0;
}
/**
* Requests a new email view from the server.
* A maximum of 20 emails can be returned.
*
* @return A new view of {@link GuerrillaMessage}s, or NULL if the response code was not OK (200) or the JSON was invalid
* @throws IOException
*/
@Nullable
public XView nextEmailList() throws IOException {
HttpMethod method = createGet("check_email");
method.getParameters().add("seq", lastEmailId);
JsonObject object = retrieve(method);
if (object == null || !object.contains("list", JsonType.ARRAY)) {
return null;
}
XList list = new XArrayList<>();
JsonArray messages = object.getArray("list");
for (Object o : messages) {
if (o instanceof JsonObject) {
JsonObject obj = (JsonObject) o;
String idStr = obj.getString("mail_id");
if (!ParseWorker.isInt(idStr)) {
XLogger.internal("Received NON-INT id '" + idStr + "'.");
continue;
}
String from = obj.getString("mail_from");
String subject = obj.getString("mail_subject");
String snippet = obj.getString("mail_excerpt");
//String timestamp = obj.getString("mail_timestamp");
boolean read = obj.getInt("mail_read") == 1;
String date = obj.getString("mail_date");
GuerrillaMessage message = new GuerrillaMessage();
message.setContent(snippet);
message.setId(ParseWorker.toInt(idStr));
message.setRead(read);
message.setSender(from);
message.setSubject(subject);
message.setWhen(LocalTime.parse(date));
list.add(message);
}
}
if (!list.isEmpty()) {
this.lastEmailId = list.last().getId();
}
return new XBasicView<>(list);
}
public void reset() throws IOException {
deleteEmail();
getCookies().setPhpSessId(null);
}
/**
* Executes the method, update the cookies according to the response,
* and constructs a JsonObject according to the response data.
*
* @param method The {@link HttpMethod}
* @return The generated {@link JsonObject}, or NULL if the response code was not OK (200)
* @throws IOException
* @see HttpMethod#execute()
* @see PlainQueryResponse
* @see #buildJson(PlainQueryResponse)
* @see #updateCookies(PlainQueryResponse)
*/
@Nullable
public JsonObject retrieve(@Nonnull HttpMethod method) throws IOException {
PlainQueryResponse response = method.execute();
if (!response.isOk()) {
return null;
}
updateCookies(response);
return buildJson(response);
}
/**
* Requests the server to respond the current user email.
* Can be used to check if the email has expired, is still valid, etc.
* Note that the email is not set to the local field.
*
* @return The new {@link GuerrillaEmail}, or NULL if the response code was not OK (200)
* @throws IOException
*/
@Nullable
public GuerrillaEmail retrieveEmail() throws IOException {
HttpMethod method = createGet("get_email_address");
method.getParameters().add("lang", "en");
JsonObject object = retrieve(method);
if (object == null) {
return null;
}
String address = object.getString("email_addr");
//String timestamp = object.getString("email_timestamp");
if (address == null) {
XLogger.internal("Received NULL address.");
}
return new GuerrillaEmail(address, LocalDateTime.now());
}
/**
* Constructs a JsonObject according to the response data.
*
* @param response The {@link PlainQueryResponse}
* @return {@link JsonObject}
* @throws IOException
*/
@Nonnull
protected JsonObject buildJson(@Nonnull PlainQueryResponse response) throws IOException {
String result = response.getContent();
JsonObject object = new JsonObject();
try {
object.load(new StringResource(result));
} catch (JsonParseException exception) {
throw new IOException(exception);
}
return object;
}
/**
* Populates the URL content of the specified properties.
*
* @param properties The properties
* @param functionName The function name to call, ex. "get_email_address"
*/
protected void populateContent(@Nonnull QueryProperties properties, @Nonnull String functionName) {
properties.add("agent", "ShuraX HTTP");
properties.add("f", functionName);
properties.add("ip", new LocalAddress().getIp());
}
/**
* Updates the local cookies according to the response header.
*
* @param response The {@link PlainQueryResponse}
* @see #getCookies()
*/
protected void updateCookies(@Nonnull PlainQueryResponse response) {
XList cookies = (XList) response.getHeader().get("Set-Cookie");
for (String cookie : cookies) {
int index = cookie.indexOf(';');
if (index != -1) {
cookie = cookie.substring(0, index);
if (cookie.startsWith("PHPSESSID=")) {
getCookies().setPhpSessId(cookie.substring(10));
} else if (cookie.startsWith("SUBSCR=")) {
getCookies().setSubscr(cookie.substring(7));
if (getCookies().getSubscr().equals("deleted")) {
getCookies().setSubscr(null);
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy