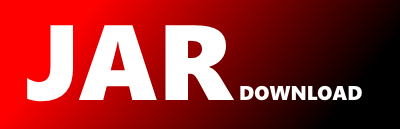
com.github.bloodshura.sparkium.mail.impl.JavaMail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sparkium-mail Show documentation
Show all versions of sparkium-mail Show documentation
An API which aims to facilitate the usage of javax.mail, also including other mailing services.
The newest version!
package com.github.bloodshura.sparkium.mail.impl;
import com.github.bloodshura.ignitium.io.File;
import com.github.bloodshura.ignitium.net.NetAddress;
import com.github.bloodshura.ignitium.sys.XSystem;
import com.github.bloodshura.ignitium.util.XApi;
import com.github.bloodshura.sparkium.mail.Mail;
import com.github.bloodshura.sparkium.mail.MailException;
import com.github.bloodshura.sparkium.mail.component.Account;
import com.github.bloodshura.sparkium.mail.component.Email;
import com.github.bloodshura.sparkium.mail.component.Message;
import com.github.bloodshura.sparkium.mail.component.Recipients;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.mail.Message.RecipientType;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.net.ssl.SSLSocketFactory;
import java.util.Properties;
public abstract class JavaMail implements Mail {
private Account account;
private ConnectionEntry connection;
private final MailService details;
private NetAddress smtp;
private int timeout;
public JavaMail() {
XApi.requireState(getClass().isAnnotationPresent(MailService.class), "No @MailService annotation found");
this.details = getClass().getAnnotation(MailService.class);
this.timeout = 8000;
}
@Override
public final void connect(@Nonnull Account account) throws MailException {
if (isConnected()) {
disconnect();
}
try {
this.connection = createConnection(account);
this.account = account;
} catch (MessagingException exception) {
throw new MailException("Failed to create connection", exception);
}
}
@Override
public final void disconnect() throws MailException {
if (isConnected()) {
this.account = null;
try {
getConnection().getTransport().close();
} catch (MessagingException exception) {
throw new MailException("Could not close connection", exception);
} finally {
this.connection = null;
}
}
}
@Nullable
@Override
public final Account getAccount() {
return account;
}
@Nullable
public final ConnectionEntry getConnection() {
return connection;
}
@Nonnull
public final MailService getDetails() {
return details;
}
@Nonnull
@Override
public final String getIdentifier() {
return getDetails().name();
}
@Nonnull
public final NetAddress getSMTP() {
if (smtp == null) {
this.smtp = new NetAddress(getDetails().address(), getDetails().port());
}
return smtp;
}
public final int getTimeout() {
return timeout;
}
@Override
public final void send(@Nonnull Recipients recipients, @Nonnull Message message) throws MailException {
if (!isConnected()) {
throw new MailException("Not connected");
}
try {
String email = getAccount().getEmail().get();
InternetAddress address = new InternetAddress(email);
MimeMessage mimeMessage = new MimeMessage(connection.getSession());
MimeMultipart mimePart = new MimeMultipart();
String content = message.getContent().toString(XSystem.getLineSeparator());
for (File attachment : message.getAttachments()) {
MimeBodyPart attachmentPart = new MimeBodyPart();
FileDataSource source = new FileDataSource(attachment.getPath());
attachmentPart.setDataHandler(new DataHandler(source));
attachmentPart.setFileName(attachment.getPath());
mimePart.addBodyPart(attachmentPart);
}
for (Email recipient : recipients.getRecipients()) {
mimeMessage.addRecipients(RecipientType.TO, InternetAddress.parse(recipient.get(), false));
}
for (Email cc : recipients.getCcRecipients()) {
mimeMessage.addRecipients(RecipientType.CC, InternetAddress.parse(cc.get(), false));
}
mimeMessage.setContent(mimePart);
mimeMessage.setFrom(address);
mimeMessage.setSubject(message.getSubject());
mimeMessage.setText(content);
connection.getTransport().sendMessage(mimeMessage, mimeMessage.getAllRecipients());
} catch (MessagingException exception) {
throw new MailException("Failed to send e-mail", exception);
}
}
public final void setTimeout(int timeout) {
this.timeout = timeout;
}
@Nonnull
protected ConnectionEntry createConnection(@Nonnull Account account) throws MessagingException {
Properties props = new Properties();
String email = account.getEmail().get();
InternetAddress address = new InternetAddress(email);
String timeout = String.valueOf(getTimeout());
props.setProperty("mail.smtp.auth", "true");
props.setProperty("mail.smtp.connectiontimeout", timeout);
props.setProperty("mail.smtp.host", getSMTP().getIp());
props.setProperty("mail.smtp.port", String.valueOf(getSMTP().getPort()));
props.setProperty("mail.smtp.quitwait", "false");
props.setProperty("mail.smtp.timeout", timeout);
props.setProperty("mail.smtp.writetimeout", timeout);
if (getDetails().sslSocket()) {
props.setProperty("mail.smtp.socketFactory.class", SSLSocketFactory.class.getName());
props.setProperty("mail.smtp.socketFactory.fallback", "false");
props.setProperty("mail.smtp.socketFactory.port", String.valueOf(getSMTP().getPort()));
}
props.setProperty("mail.smtp.starttls.enable", String.valueOf(getDetails().ssl()));
Session session = Session.getInstance(props);
Transport transport = session.getTransport(address);
transport.connect(getSMTP().getIp(), /*getSMTP().getPort(),*/ email, account.getPassword().toString());
return new ConnectionEntry(session, transport);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy