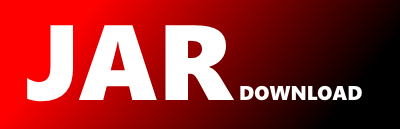
cn.bluejoe.xmlbeans.node.value.BeanEntityNode Maven / Gradle / Ivy
package cn.bluejoe.xmlbeans.node.value;
import java.util.ArrayList;
import java.util.List;
import org.dom4j.Element;
import cn.bluejoe.xmlbeans.node.PropertyNode;
import cn.bluejoe.xmlbeans.node.XmlSerializableNode;
/**
* @author [email protected]
*/
public class BeanEntityNode extends AbstractXmlNode implements XmlSerializableNode, ValueNode, EntityNode
© 2015 - 2025 Weber Informatics LLC | Privacy Policy