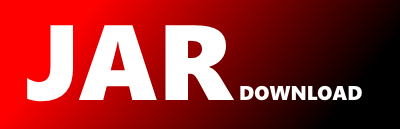
com.github.bookong.zest.util.DateUtils Maven / Gradle / Ivy
package com.github.bookong.zest.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
/**
* @author jiangxu
*
*/
public class DateUtils {
private static final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy