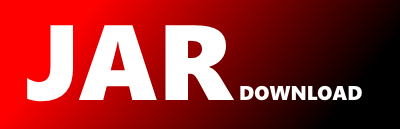
com.github.bookong.zest.util.SqlHelper Maven / Gradle / Ivy
package com.github.bookong.zest.util;
import java.math.BigDecimal;
import java.sql.Connection;
import java.sql.NClob;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author jiangxu
*
*/
public class SqlHelper {
public static void safeClose(Statement stat) {
if (stat != null) {
try {
stat.close();
} catch (SQLException e) {
System.out.println("Fail to close Statement.");
}
}
}
public static void safeClose(ResultSet rs) {
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
System.out.println("Fail to close ResultSet.");
}
}
}
/** 从数据库中捞取数据并做适当的转换后返回 */
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy