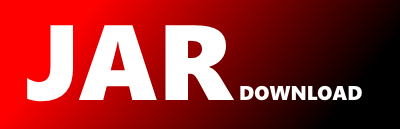
com.github.brainlag.nsq.netty.NSQDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nsq-client Show documentation
Show all versions of nsq-client Show documentation
Fast Java client for NSQ.
The newest version!
package com.github.brainlag.nsq.netty;
import com.github.brainlag.nsq.frames.NSQFrame;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.MessageToMessageDecoder;
import java.util.List;
public class NSQDecoder extends MessageToMessageDecoder {
private int size;
private NSQFrame frame;
public NSQDecoder() {
}
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy