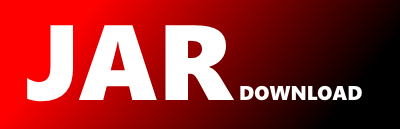
com.github.brainlag.nsq.netty.NSQEncoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nsq-client Show documentation
Show all versions of nsq-client Show documentation
Fast Java client for NSQ.
The newest version!
package com.github.brainlag.nsq.netty;
import com.github.brainlag.nsq.NSQCommand;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.MessageToMessageEncoder;
import java.util.List;
public class NSQEncoder extends MessageToMessageEncoder {
@Override
protected void encode(ChannelHandlerContext ctx, NSQCommand message, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy