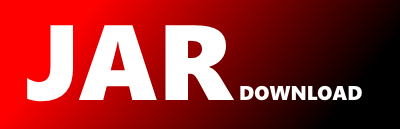
com.bumptech.glide.load.engine.LoadPath Maven / Gradle / Ivy
package com.bumptech.glide.load.engine;
import android.support.v4.util.Pools.Pool;
import com.bumptech.glide.load.Options;
import com.bumptech.glide.load.data.DataRewinder;
import com.bumptech.glide.util.Preconditions;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* For a given {@link com.bumptech.glide.load.data.DataFetcher} for a given data class, attempts to
* fetch the data and then run it through one or more
* {@link com.bumptech.glide.load.engine.DecodePath}s.
*
* @param The type of data that will be fetched.
* @param The type of intermediate resource that will be decoded within one of the
* {@link com.bumptech.glide.load.engine.DecodePath}s.
* @param The type of resource that will be returned as the result if the load and
* one of the decode paths succeeds.
*/
public class LoadPath {
private final Class dataClass;
private final Pool> listPool;
private final List extends DecodePath> decodePaths;
private final String failureMessage;
public LoadPath(Class dataClass, Class resourceClass,
Class transcodeClass,
List> decodePaths, Pool> listPool) {
this.dataClass = dataClass;
this.listPool = listPool;
this.decodePaths = Preconditions.checkNotEmpty(decodePaths);
failureMessage = "Failed LoadPath{" + dataClass.getSimpleName() + "->"
+ resourceClass.getSimpleName() + "->" + transcodeClass.getSimpleName() + "}";
}
public Resource load(DataRewinder rewinder, Options options, int width,
int height, DecodePath.DecodeCallback decodeCallback) throws GlideException {
List exceptions = listPool.acquire();
try {
return loadWithExceptionList(rewinder, options, width, height, decodeCallback, exceptions);
} finally {
listPool.release(exceptions);
}
}
private Resource loadWithExceptionList(DataRewinder rewinder, Options options,
int width, int height, DecodePath.DecodeCallback decodeCallback,
List exceptions) throws GlideException {
int size = decodePaths.size();
Resource result = null;
for (int i = 0; i < size; i++) {
DecodePath path = decodePaths.get(i);
try {
result = path.decode(rewinder, width, height, options, decodeCallback);
} catch (GlideException e) {
exceptions.add(e);
}
if (result != null) {
break;
}
}
if (result == null) {
throw new GlideException(failureMessage, new ArrayList<>(exceptions));
}
return result;
}
public Class getDataClass() {
return dataClass;
}
@Override
public String toString() {
return "LoadPath{" + "decodePaths="
+ Arrays.toString(decodePaths.toArray(new DecodePath[decodePaths.size()])) + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy